Tkinter Grid
Hey there!
So you’ve started playing around with Python and GUI apps using Tkinter? Awesome!
One of the coolest things you’ll need to learn is how to arrange your widgets on the screen.
And that’s where grid()
comes in.
Let’s dive in. I promise it’s going to be easy and kind of fun. 😄
In the previous article, we discussed pack() check it out if you haven’t already.
Contents
Tkinter Grid
What is grid() in Tkinter?
Okay, imagine your app is like a spreadsheet. You’ve got rows and columns.
With grid()
, you can tell Python:
“Hey, I want this button in row 1, column 0.”
And boom! It lands right there.
💡 Use
grid()
when you want to line things up neatly. It’s perfect for forms, calculators, and stuff with a nice layout.
Tkinter Grid Tkinter Grid
Setting Up a Basic Tkinter Window
Before we use grid, let’s set up a tiny window:
import tkinter as tk
root = tk.Tk()
root.title("My Cool App")
Let’s Add Some Widgets with grid()
Now let’s add some stuff. A label, an entry, and a button:
label = tk.Label(root, text="Your Name:")
label.grid(row=0, column=0)
entry = tk.Entry(root)
entry.grid(row=0, column=1)
button = tk.Button(root, text="Say Hello")
button.grid(row=1, column=0, columnspan=2)
root.mainloop()
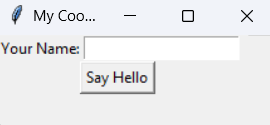
What just happened?
- The label goes in row 0, column 0.
- The entry goes in row 0, column 1.
- The button goes in row 1, but it stretches across two columns!
Tkinter Grid Tkinter Grid
More Cool Stuff You Can Do with grid()
Spacing it Out
Want some space around your widgets?
label.grid(row=0, column=0, padx=10, pady=5)
padx
adds space left/right.pady
adds space top/bottom.
Stick It!
Widgets by default sit in the middle. But you can “stick” them to a side.
label.grid(row=0, column=0, sticky="w")
"w"
= west (left)"e"
= east (right)"n"
= north (top)"s"
= south (bottom)
Using grid() with Loops
This is where things get fun. Let’s make a 3×3 button grid!
for row in range(3):
for col in range(3):
btn = tk.Button(root, text=f"({row},{col})")
btn.grid(row=row, column=col)
Boom! Nine buttons lined up perfectly.
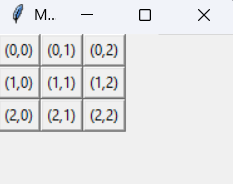
Mixing grid() and pack()? Don’t!
Just a quick warning:
Never mix grid()
and pack()
in the same container.
Your app might go bonkers. Stick to one layout method per frame.
Wrap Up: Why You’ll Love grid()
- It’s simple and predictable.
- It helps you create clean layouts.
- And once you get the hang of rows and columns—you’re golden!
You now know enough to build forms, dashboards, and even games with layout power.