Tkinter Text Widget With Example
Hey friend! 👋
Today, we’re talking about something super cool in Tkinter: the Text widget.
Think of it like a supercharged version of Entry
.
You can type a lot more stuff. Multi-line, rich text, even images. Yup—it’s that powerful.
🎯 Sneak Peek:
Tomorrow, we’ll build a full Rich Text Editor with bold, italic, font styles, colors, links—you name it. But first, let’s master the basics.
Ready? Let’s make this widget your new best friend. 👇
Contents
Introduction to the Tkinter Text Widget
The Text
widget is a multi-line text area.
You can write, read, and play around with text. It’s perfect for:
- Notes
- Chat windows
- Code editors
- Anything text-heavy
It’s super customizable, and once you get the hang of it—you’ll be hooked.
Creating a Simple Text Widget
Here’s the basic setup:
import tkinter as tk
root = tk.Tk()
root.title("Text Widget Demo")
root.geometry("400x300")
text = tk.Text(root)
text.pack(pady=20, padx=20)
root.mainloop()
You now have a blank box where users can type anything.
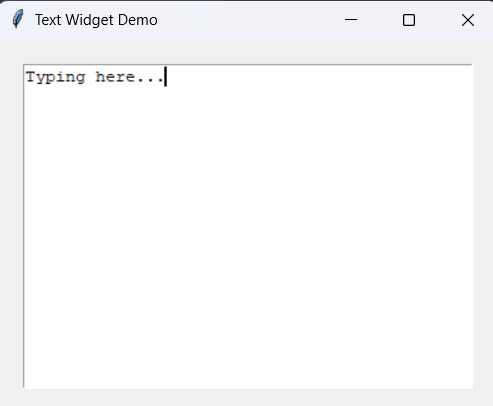
Adding Content to the Text Widget
Want to add some starting text? Easy:
text.insert("1.0", "Hello there!\nThis is a Tkinter Text widget.")
Tkinter Text Widget With Example
"1.0"
means line 1, character 0 (the very beginning).
You can insert text anywhere you want by changing the line/column.
You can remove this text and type in here too.
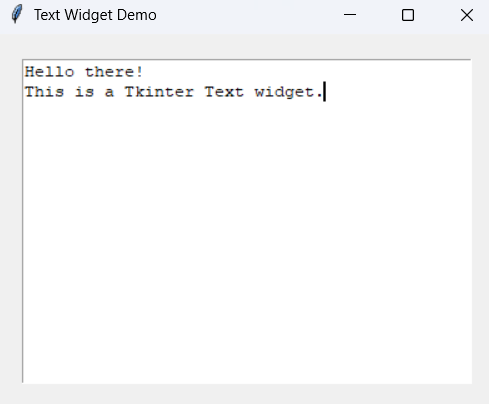
Retrieving Text from the Text Widget
Need to get what someone typed? Use this:
content = text.get("1.0", "end-1c")
print(content)
"1.0"
is the start
"end-1c"
means one character before the end (to avoid the extra newline)
Tkinter Text Widget With Example Tkinter Text Widget With Example
Disabling and Enabling the Text Widget
Sometimes you want to lock it—like for displaying only:
text.config(state="disabled")
And if you want to let users type again:
text.config(state="normal")
You can directly provide state too. In the widget itself.
Deleting Contents from the Text Widget
Clear it all out like this:
text.delete("1.0", "end")
Bye-bye, text! You can also delete a range if needed.
Changing the Appearance of the Text Widget
You can style it like this:
text.config(font=("Arial", 14), fg="darkblue", bg="lightyellow", height=10, width=40)
Tkinter Text Widget With Example
font
: set the font type and sizefg
: text colorbg
: background colorheight
andwidth
: control the size
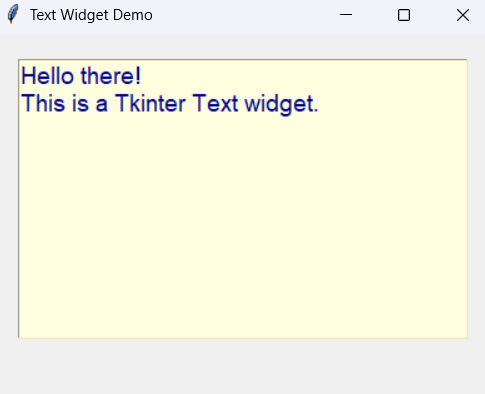
Embedding Links (Sort of)
The Text
widget doesn’t have real HTML-style links,
but you can make clickable text using tags:
def clicked(event):
print("Link clicked!")
text.insert("1.0", "Click here!")
text.tag_add("link", "1.0", "1.10")
text.tag_config("link", foreground="blue", underline=True)
text.tag_bind("link", "<Button-1>", clicked)
Now “Click here!” looks like a link—and works like one too.
Embedding Images in the Text Widget
Yes, you can even add images!
from tkinter import PhotoImage
photo = PhotoImage(file="ocean.png")
text.image_create("end", image=photo)
Remember: you must keep a reference to the image (like storing it in a variable), or it won’t show up!
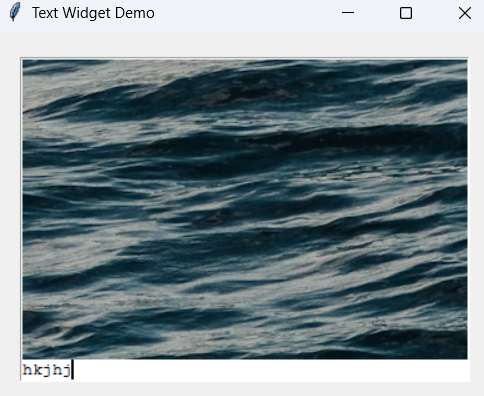
💡Challenge: Build Your Own Rich Text Editor with Tkinter!
Alright, you’ve learned the magic of the Text
widget—
But are you ready to level up?
Here’s a challenge for you (yup, I dare you 😄):
Build a basic text editor with these features:
- Bold selected text
- Italic selected text
- Underline selected text
- Change font size
- Change font family
- Change font color
- Add a clickable link
- Clear text area
- Save and open a
.txt
file (bonus) (if you know files in Python)
Tkinter Text Widget With Example Tkinter Text Widget With Example