Have you heard of the word anonymous writer? This means that we don’t know the writer’s name.
Lambda functions in python are pretty similar as they don’t have a name.
Let’s explore what are these:
- mysterious objects or
- simple functions to make python a bit easier…
Contents
Previous post’s challenge’s solution
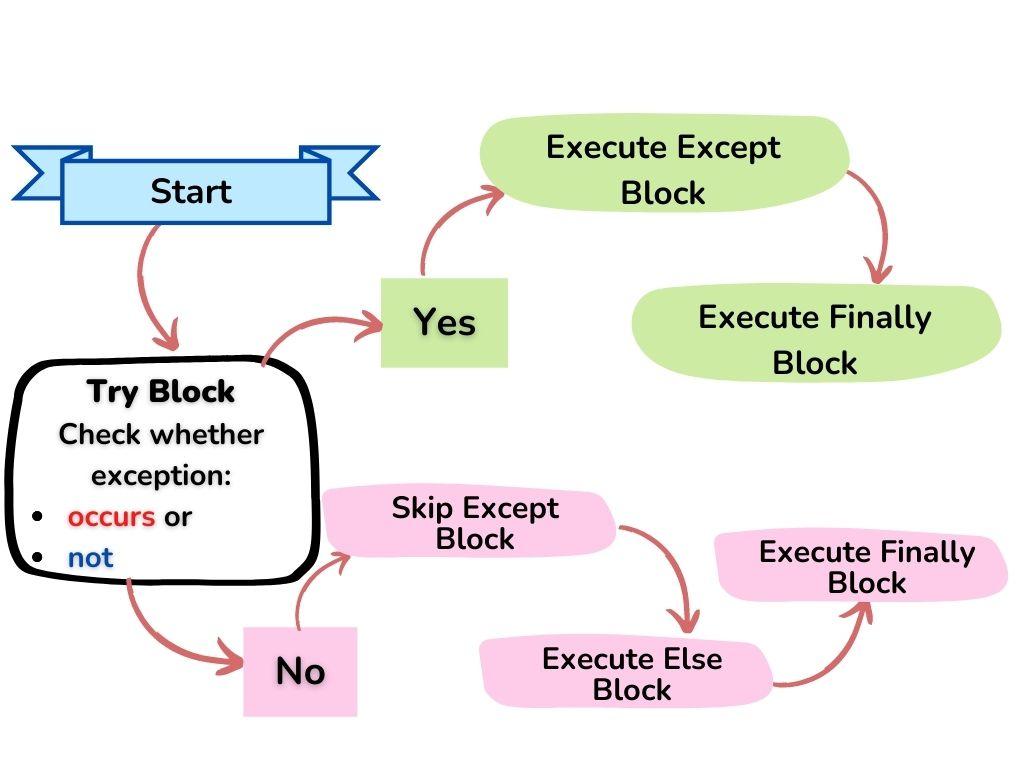
What are lambda functions?
These functions are:-
- One liner;
- Can have multiple🤹♀️arguments;
- Only one👆expression;
- No name🤫;
- Defined using the
lambda
keyword.
This is how to define one:
sqaure = lambda n: n*n
print(square(10))
Output:
100
This is a simple function that takes a number n
as an argument and then returns its square.
NOTE: This is not the correct way to use this function it’s discouraged by PEP8.
It would be helpful in case your teacher👩🏫 suddenly starts asking you all squares of random numbers🔢.
It will give you a good impression😎 among your friends and save you from the teacher’s👩🏫 punishment.
Syntax:
First, the keyword lambda
, followed by arguments
, then a colon
, and finally the expression
.
lambda argument(s): expression
Are you thinking🤔 something like “why on the earth🌏 did you not tell me this before”? It is much simpler than defining functions using the def
keyword like we have been doing earlier.
But trust me using these functions isn’t a great idea💡 always.
They are great🎉 for some uses and to be avoided🙅♀️ for some.
Let’s see 👀 when and when not to use them.
When to use and when not to use?
When to use them
- When you want to pass them to some high-order function like the map function.
A high-order function is the one which:
- takes a function as an argument or;
- returns a function
- When you want to use a function temporarily. Maybe for testing or anything like that. ( Or maybe you have mood swings😅)
- When you need just one or even no expression in the function.
- When you want to use the function just once.
When not to use them
- When you need to name a lambda function. PEP8, the official Python style guide, advises never to write code like this.
- Whenever you want to give a single argument taking function inside of it:
numbers = [10,2,-343,25,-12]
sorted_numbers = sorted(numbers, key=lambda n: abs(n))
print(sorted_numbers) #[2, 10, -12, 25, -343]
The abs()
function takes a number as its argument and returns its absolute value. (Converts a number to its positive)
Here we sorted the sequence of numbers based on their absolute value, we don’t care about their being positive or negative.
We could have directly given the abs function inside the key argument of the sorted function. We unnecessarily used the lambda function here.
- When using multiple lines would make the code more readable. Like while using if else inside the function.
You can read this article if you want to know more about where not to use them.
map function
The Map is an inbuilt function in python. It takes a function and an iterable(s) as its arguments.
After that, it applies the function to all the elements of that iterable and returns a map object
.
This can later be converted to a list/tuple or any other iterable.
This is the most common use case of the lambda function.
Say for example you want to multiply all the elements of a list to the world’s most favorite number 7.
Here’s how you can do it using the map and lambda function:
nums = [10, 12, 32]
multiplied_by_seven = map(lambda x: x * 7, nums)
# multiplied_by_seven is a variable that stores a map object.
# The lambda function takes a number as argument and returns its
# multiplication with 7.
# The map function applies this lambda function to all the
# elements of the list.
# multiplied_by_seven is a map object.
# You can check this by printing its type.
# Converted the map object into a list
num_list = list(multiplied_by_seven)
print(num_list)
Output:
[70, 84, 224]
A misunderstanding:
The map is a class
in python3 it used to be a function
in python2. However, the documentation is not updated mentioning this fact.
If you want to read about this in greater detail I suggest reading this StackOverflow answer.
The same is the case with the filter function that we are going to discuss next.
Filter function
The filter function as the name might suggest🤔 is proper when you want to filter out certain elements from a list.
The Filter is also an inbuilt function in python. It takes a function and an iterable(s) as its arguments.
Say for example you have a large number of movies🎞 on your system🖥.
Now you have to delete some of them as your hard drive is full🤢 and you cannot afford💵 a new one right now.
There are certain movies in your system that you have watched more than thrice🥱. And you have at least watched all the movies twice. You’re thinking🤔 it would be ok to delete the movies🎞 you watched more than twice.
So for this, you copied all the movie names in a list along with the number of times you have watched them:
movies = ["Star Wars: The Force Awakens 4", "Avengers: Endgame 2",
"Spider-Man: No Way Home 6", "Avatar 2", "Black Panther 5"]
Then by using the filter function you filtered out the movies🎞.
The lambda function will return the string(movie🎞) if it ends with 2.
This is because you have decided to keep all movies🎞 that you have watched twice.
movies_left = filter(lambda x: x.endswith("2"), movies)
print(list(movies_left))
Now finally you are left with movies🎞 that you have watched just twice.
It feels great to clean up😅. You might go to your friends and brag: “Less is More.”😜🤣
['Avengers: Endgame 2', 'Avatar 2']
Let’s now move on to the reduce
function from the functools
library.
Reduce function
This function takes a function and an iterable(s) as arguments. It reduces the iterable to a single value.
Say, for example, you and your friends want to know who is the tallest. But because the height differences are minor you are unable to tell who is tallest among you all.
So you decide to store all the heights in a list. And use the reduce function on them to know who’s the tallest:
from functools import reduce
heights = [162, 155, 170, 159, 168, 171]
tallest = reduce(lambda x, y: x if x>y else y, heights)
print(tallest)
Output:
171
- Here, first, you used reduce function as you wanted just one value as a result.
- Next, you used a lambda function that takes two arguments x and y, and returns the value which is greater among them.
- That’s how we got the largest number from the list.
- As a result, we got to know who is the tallest.
This might seem like something really stupid but it would be highly useful when you are dealing with hundreds and thousands of numbers.
Conclusion
In this post, we saw that lambda functions are not mysterious objects. They are one-liner functions in python.
Then we saw that they are easier to use compared to normal functions that we define using the def keyword. But, their use is not recommended everywhere.
After that, we saw the use of the lambda function in 3 high-order functions.
We took some simple examples to understand this.
Challenge🧗♀️
Your challenge for this post is to make a typing speed tester in python. Don’t be scared by this time it is super easy for you.
Hints/starting steps:-
- You’ll need to use the time module to calculate speed.
- You might divide your program into functions for convenience.
- You’ll also need to match the strings.
- speed = distance(words)/time.
You don’t need to create anything high-fi with GUIs. Just a simple typing speed calculator.
Go fast. I am waiting. Comment your answers below.
This was it for the post. Comment below suggestions if there and tell me whether you liked it or not. Do consider sharing this with your friends.
See you in the next post till then have a great time.😊