Ok so by now you have come across a number of data types in python including integers, float, strings, lists, dictionaries, boolean, and complex numbers. Today in this post for the first time we will explore an immutable datatype which is tuples.
Tuple in python official documentation.
Contents
Previous post’s challenge’s solution
money = """
___________________________________
|#######====================#######|
|#(1)*UNITED STATES OF AMERICA*(1)#|
|#** /===\ ******** **#|
|*# {G} | (") | #*|
|#* ****** | /v\ | O N E *#|
|#(1) \===/ (1)#|
|##=========ONE DOLLAR===========##|
------------------------------------
"""
print(money)
price_profit_revenue = {"apple": [100, 10000, 1000],
"banana": [90, 9000, 900],
"spinach": [50, 4000, 800],
"pineapples": [100, 8000, 900]}
print(price_profit_revenue)
Output:-
___________________________________
|#######====================#######|
|#(1)*UNITED STATES OF AMERICA*(1)#|
|#** /===\ ******** **#|
|*# {G} | (") | #*|
|#* ****** | /v\ | O N E *#|
|#(1) \===/ (1)#|
|##=========ONE DOLLAR===========##|
------------------------------------
{'apple': [100, 10000, 1000], 'banana': [90, 9000, 900], 'spinach': [50, 4000, 800], 'pineapples': [100, 8000, 900]}
I think the code and output are self-explanatory but comment below if you have a doubt.
What are tuples?
Tuples are pretty similar to lists except for the facts:
- They are enclosed in round brackets
'()'
; - They are immutable.
Immutable is a fairly new word to you, right? But, it simply means that you cannot change the data inside of a tuple in any given way, the example below will make the meaning of immutable clear to you:-
tup1 = (10, 20, 30, 50)
tup1[3] = 40
print(tup1)
Output:-
Traceback (most recent call last):
File "c:\Desktop\python-hub.com\tup.py", line 2, in
tup1[3] = 40
TypeError: 'tuple' object does not support item assignment
However, you can:
- Concatenate(combine/join) two or more tuples to form a new tuple;
- Can also delete a tuple completely once created;
- And, can create a copy of the tuple.
We will take a look at all these in this section.
Ways To Create One
There are mainly 3 ways of creating them:
- One that you saw above by enclosing the data into parenthesis
'()'
- The second is using the tuple function:-
homework = tuple(["Multiplication Table of Two", \
"A poem on environment", "Process of Photosynthesis"])
print(homework)
print(type(homework))
Output:-
('Multiplication Table of Two', 'A poem on environment', 'Process of Photosynthesis')
<class 'tuple'>
- The third one is by giving data in comma-separated sequence:-
homework = "Multiplication Table of Two", \
"A poem on environment", "Process of Photosynthesis"
print(homework)
print(type(homework))
Output:-
('Multiplication Table of Two', 'A poem on environment', 'Process of Photosynthesis')
<class 'tuple'>
Single value tuple
These can prove to be quite tricky. See the example:-
s_tup = "hello" # This will be a string
s1_tup = "hello", # This will be a tuple
s2_tup = ("hello") # This will be a string
s3_tup = ("hello",) # This will be a tuple
print(f"s_tup = {s_tup} {type(s_tup)}")
print(f"s1_tup = {s1_tup} {type(s1_tup)}")
print(f"s2_tup = {s2_tup} {type(s2_tup)}")
print(f"s3_tup = {s3_tup} {type(s3_tup)}")
Output:-
s_tup = hello <class 'str'>
s1_tup = ('hello',) <class 'tuple'>
s2_tup = hello <class 'str'>
s3_tup = ('hello',) <class 'tuple'>
So, for making a tuple you need to enclose it inside a round bracket and also need to put values in a comma-separated sequence. Even if it has only one value.
Can’t understand why there’s an f before the string click here. And to read more on strings click here.
Accessing And Slicing Tuples
I suggest randomly practicing with different values to get a crystal clear understanding of the topic.
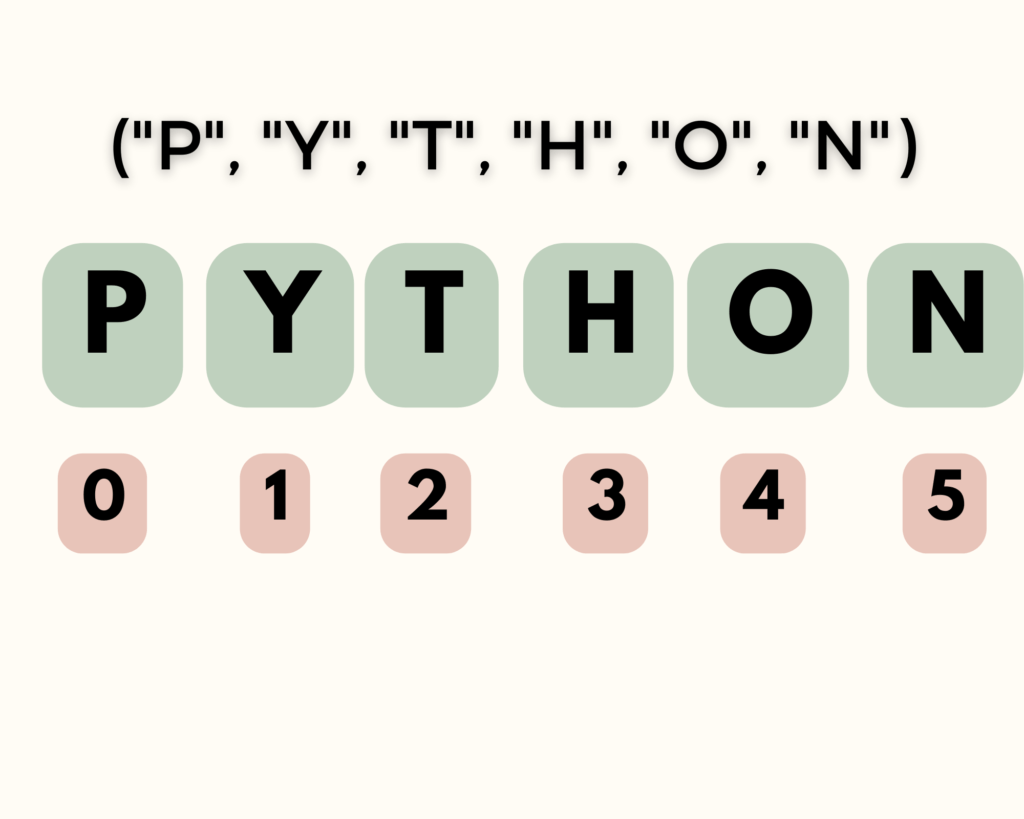
Accessing
This includes having an access to individual elements in a tuple. Just like most of the other sequence data types in python like strings and lists indexes start from 0 in a tuple too. (See the figure above)
You can simply access any element in a tuple like this:-
homework = ("Multiplication Table of Two", \
"A poem on environment", "Process of Photosynthesis")
print(homework[1])
Output:-
A poem on environment
If you give an index number that isn’t present in the tuple you will get an IndexError:-
homework = ("Multiplication Table of Two", \
"A poem on environment", "Process of Photosynthesis",\
"Maps of the world", "glorious past revision")
print(homework[5])
Output:-
Traceback (most recent call last):
File "c:\Desktop\python-hub.com\tup.py", line 5, in
print(homework[5])
IndexError: tuple index out of range
Pretty easy right especially if you have done it correctly for strings and lists.
Slicing
Slicing is using a part of the tuple. It is also super easy. You just need to follow the syntax:- tuple[start_index : stop_index : step_index]
homework = ("Multiplication Table of Two", \
"A poem on environment", "Process of Photosynthesis",\
"Maps of the world", "glorious past revision")
print(homework[1:4:2])
Output:-
('A poem on environment', 'Maps of the world')
using negative indexes
Negative indexes can be used for both accessing and slicing. Look at the figure above in that figure n
will have the index number as -1
, o
will have -2
, h
will have -3
, and so on. Here’s an example of how.
homework = ("Multiplication Table of Two", \
"A poem on environment", "Process of Photosynthesis",\
"Maps of the world", "glorious past revision")
print()
print(homework[::-1]) #reverses the list -1 is taken as a step
here and whole tuple is to be accessed
print()
print(homework[-2])
Output:-
('glorious past revision', 'Maps of the world', 'Process of Photosynthesis', 'A poem on environment', 'Multiplication Table of Two')
Maps of the world
Deleting Tuples
Deleting a tuple is very easy just type the del
keyword and then the tuple's
name.
homework = ("Multiplication Table of Two", \
"A poem on environment", "Process of Photosynthesis",\
"Maps of the world", "glorious past revision")
del homework
print(homework)
Output:-
Traceback (most recent call last):
File "c:\Desktop\python-hub.com\tup.py", line 6, in
print(homework)
NameError: name 'homework' is not defined
✨For the ones who are confused🤔🤯 :- Immutable means you cannot change any item inside the tuple but you can delete the entire tuple it has nothing to do with immutability.✨
Other Operations And Methods On Tuples
Time to use different operations and methods on tuples.
Operations On Tuples
Concatenate
You can easily join two tuples and assign them to another variable. See below:-
homework1 = ("Multiplication Table of Two", \
"A poem on environment", "Process of Photosynthesis")
homework2 = ("Maps of the world", "glorious past revision")
homework = homework1 + homework2
print(homework)
Output:-
('Multiplication Table of Two', 'A poem on environment', 'Process of Photosynthesis', 'Maps of the world', 'glorious past revision')
Copy
For creating a copy of a tuple simply assign it to another variable.
homework = ("Multiplication Table of Two", \
"A poem on environment", "Process of Photosynthesis",\
"Maps of the world", "glorious past revision")
print(homework)
HW = homework
print(HW)
Output:-
('Multiplication Table of Two', 'A poem on environment', 'Process of Photosynthesis', 'Maps of the world', 'glorious past revision')
('Multiplication Table of Two', 'A poem on environment', 'Process of Photosynthesis', 'Maps of the world', 'glorious past revision')
Methods On Tuples
Count()
Suppose your teacher 👩🏫 by mistake gave you the same homework more than once and you want to count the number of times it has been given to point out her mistake. This is where the count function helps you.
homework = ("Multiplication Table of Two", \
"A poem on environment", "Multiplication Table of Two",\
"Process of Photosynthesis",\
"Maps of the world", "glorious past revision",\
"Multiplication Table of Two")
print(homework)
print()
print(homework.count("Multiplication Table of Two"))
Output:-
('Multiplication Table of Two', 'A poem on environment', 'Multiplication Table of Two', 'Process of Photosynthesis', 'Maps of the world', 'glorious past revision', 'Multiplication Table of
Two')
3
index()
It is used to find out the index number of an element inside the tuple. If more than one occurrence of the element is there in the tuple then the index of the first occurrence is shown
homework = ("Multiplication Table of Two", \
"A poem on environment", "Multiplication Table of Two",\
"Process of Photosynthesis",\
"Maps of the world", "glorious past revision",\
"Multiplication Table of Two")
print(homework)
print()
print(homework.index("Multiplication Table of Two"))
Output:-
('Multiplication Table of Two', 'A poem on environment', 'Multiplication Table of Two', 'Process of Photosynthesis', 'Maps of the world', 'glorious past revision', 'Multiplication Table of
Two')
0
Conclusion
In this post, we for the first time saw an immutable data type tuple. Also, we saw different ways to create a tuple, and it’s accessing and slicing. Along with this, we saw some operations and methods on tuples.
Challenge🧗♀️
Now your challenge is to help your class teacher👩🏫 to store the names and 📚homework-done(minimum of 3 subjects) details of all the students(minimum of 3).
Names should be stored in a dictionary and 📚homework done details should be stored in a tuple. You are required to use a nested dictionary for this. It is nothing more than a fancy way of saying tuple/list/dictionary/any data type inside a dictionary.
Go fast. I am waiting. Comment your answers below.
This was it for the post. Comment below suggestions if there and tell me whether you liked it or not. Do consider sharing this with your friends.
See you in the next post till then have a great time.😊
This site truly has all the information I needed concerning this subject and didn’t know who to ask.
Thanks a lot Talitha Ciskowski. It’s a pleasure to know it helped you. Stay tuned for more such content on python programming