Hello techies welcome back
Today let’s start with building the foundation of the ChillPill. We would first need to know the folder structure of Flutter.
Contents
There are 2 popular ways to structure a flutter project in the community. However, flutter being flexible doesn’t make any structure compulsory.
- Layer based structuring
- Feature-based structuring
What is the basic folder structure of Flutter?
Under the first one, we put the pages into directories based on the layer they belong to. You can consider this the MVC(model view control) way.
For example, we are creating a chat feature.
The UI of this feature would be in one directory, the backend logic functions in another, and database-related logic in another.
What is feature first architecture in Flutter?
Under the feature-based structure, all the files of one feature stay under one directory.
Everything related to the chat feature would be present in one folder. Everything related to authentication will be in another. And so on.
The first one is a great way for small projects.
And the second is a great one for larger ones.
I would go with the second one as it is more reusable too(for me).
Selecting a Theme
First, let’s start with a theme design.
I suck at designs so I rushed to Google for a color palette idea.
After a few searches, I landed on this one:
https://aicolors.co/ (not sponsored)
On this website, I found 2 very good color palettes. One for light theme and one for dark theme.
Here are they:
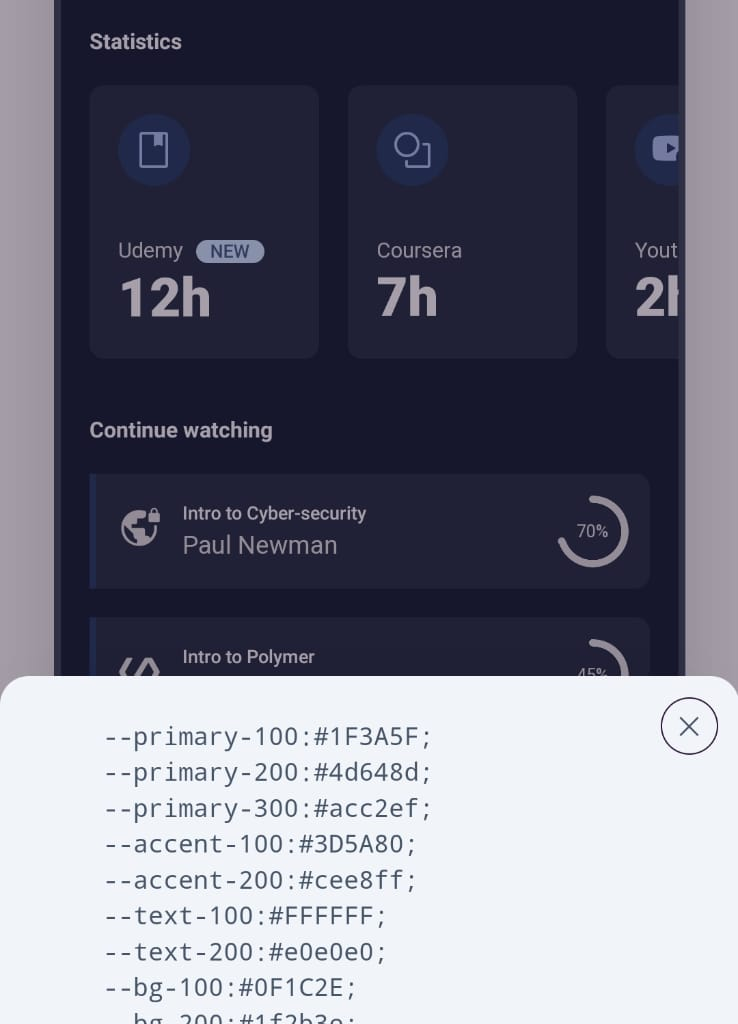
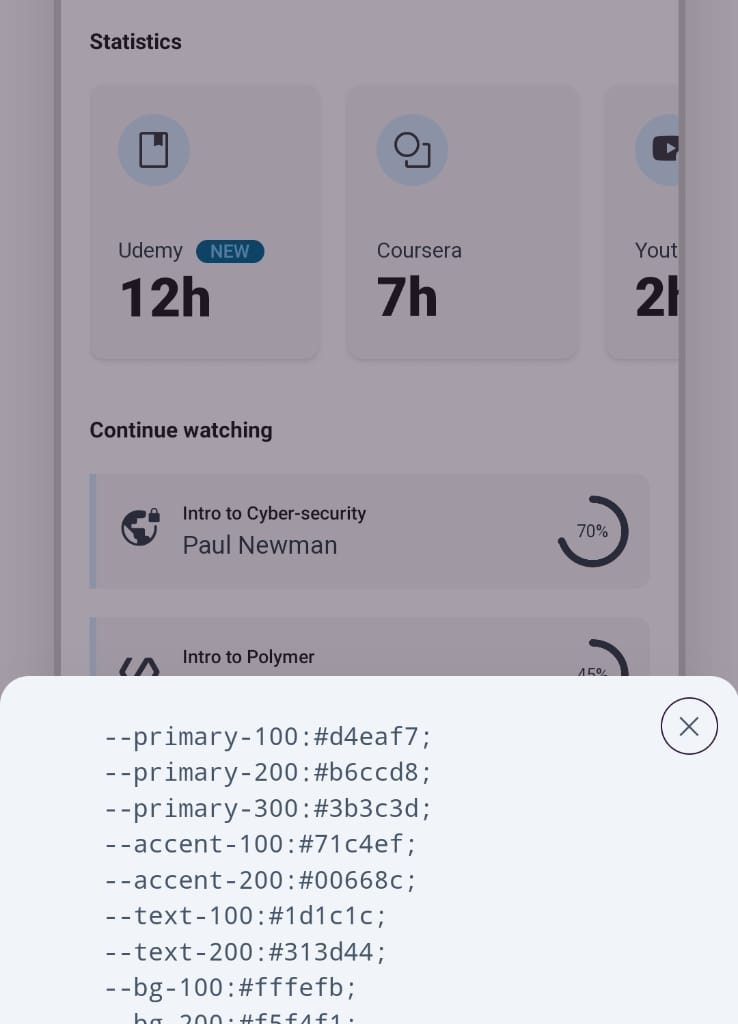
Color Codes:
//Dark theme
primary-100: #1f3a5f (dark shade)
primary-200: #4d648d (light shade)
primary-300: #acc2ef (lighter shade)
accent-100: #3d5a80
accent-200: #cee8ff
text-100: #ffffff
text-200: #e0e0e0
bg-100: #0f1c2e
bg-200: #1f2b3e
bg-300: #374357
//Light theme
primary-100: #d4eaf7
primary-200: #b6ccd8
primary-300: #3b3c3d
accent-100: #71c4ef
accent-200: #00668c
text-100: #1d1c1c
text-200: #313d44
bg-100: #fffefb
bg-200: #f5f4f1
bg-300: #cccbc8
How do you display an image file in Flutter?
The first folder/directory that we will create is assets. It would contain all the images, logos, fonts, icons, etc we want to include in our Flutter app.
Create the asset folder here:
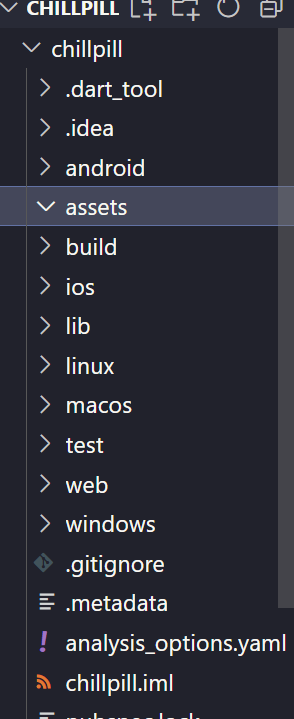
Now inside this create a folder:
- images
We currently don’t have anything but later on, we will need these assets as our app grows. Assets hold a lot more than images.
And now add this folder in the pubspec.yaml as:
assets/images/
Because we want to access everything inside the image.
And here’s how you display an image:
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(title),
),
body: Image.asset("assets/images/sample_image.jpg"),
);
}
}
folder structure of Flutter folder structure of Flutter
And that’s it for today. We will continue building ChillPill in the next video.