Dice Simulator in Python
Imagine this: it’s a cold night, and you’re all set to play Ludo with your family.
But every time you need to roll the dice, it means pulling your hands out of those warm pockets – not exactly tempting!
And if you play on your phone, your little sister has a way of sneaking in some creative moves.
So, here’s a perfect solution: a dice simulator in Python!
And it comes with a twist – landing on a six is just a bit trickier, adding some suspense to the game.
Now you can keep your hands toasty, let the dice roll and best of all… no more sneaky cheats!
Contents
Dice Simulator in Python
What you’ll build:
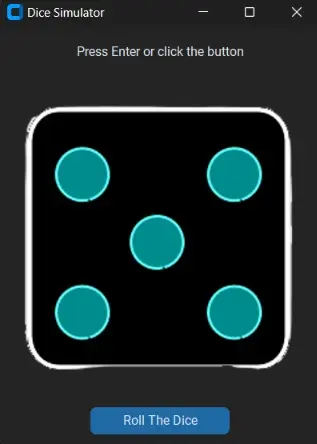
Step 1: Import Required Libraries
Start by importing the necessary libraries for this project.
from customtkinter import *
import random
from PIL import Image
Step 2: Create the Dice
Class
This class will initialize the GUI window and set up the dice simulator app.
- Define the
Dice
class, inheriting fromCTk
to create a customtkinter window. - Set the window title in the
__init__
method.
class Dice(CTk):
def __init__(self):
super().__init__()
self.title("Dice Simulator")
Step 3: Load Dice Images
- Use the
CTkImage
class to load six dice images. - Each image represents a different face of the dice.
- Set the
size
argument to control the image display size in the GUI.
# Load dice images
self.dice1 = CTkImage(light_image=Image.open("images/dice1.png"), size=(300, 300))
self.dice2 = CTkImage(light_image=Image.open("images/dice2.png"), size=(300, 300))
self.dice3 = CTkImage(light_image=Image.open("images/dice3.png"), size=(300, 300))
self.dice4 = CTkImage(light_image=Image.open("images/dice4.png"), size=(300, 300))
self.dice5 = CTkImage(light_image=Image.open("images/dice5.png"), size=(300, 300))
self.dice6 = CTkImage(light_image=Image.open("images/dice6.png"), size=(300, 300))
Step 4: Create an Image List
- We want to make it harder to roll a 6, so we modify the
image_list
. - Add images for dice 1 to 5 twice, and only add dice 6 once, reducing the likelihood of rolling a 6.
# image list to make rolling a 6 tougher
self.image_list = [self.dice1, self.dice2, self.dice3, self.dice4, self.dice5,
self.dice1, self.dice2, self.dice3, self.dice4, self.dice5,
self.dice6] # 6 appears only once
Step 5: Add an Information Label
- Create a label at the top of the window to display instructions to the user.
- Use
grid
to set the label’s position within the window.
# Label for information
label = CTkLabel(self, text="Press Enter or click the button")
label.grid(row=0, padx=10, pady=10)
Step 6: Add a Button to Roll the Dice
- Add a button that allows users to “roll” the dice by clicking it.
- Connect the button to the
roll
method, which will display a random dice face.
# Button to roll the dice
btn = CTkButton(self, text="Roll The Dice", command=self.roll)
btn.grid(row=2, padx=10, pady=10)
Step 7: Connect the Enter Key to the Button
- Use the
bind
method to connect the Enter key to the button, so users can roll the dice by pressing Enter as well. - This is done by invoking the button’s action when the Enter key is pressed.
# Connect button to the Enter key
self.bind("<Return>", lambda event: btn.invoke())
Step 8: Define the roll
Method
- In the
roll
method, randomly choose an image fromimage_list
usingrandom.choice
. - Create a label that displays the chosen dice image.
- Use
grid
to position the image label within the window.
def roll(self):
# Choose a random die face with modified probabilities
img = random.choice(self.image_list)
# Display selected die face
self.label = CTkLabel(self, text="", image=img)
self.label.grid(row=1, padx=10, pady=10)
Step 9: Run the Application
Set up the Dice
instance and call mainloop
to display the window and start the app.
if __name__ == "__main__":
app = Dice()
app.mainloop()
Dice Simulator in Python Dice Simulator in Python Dice Simulator in Python Dice Simulator in Python Dice Simulator in Python
Ending Challenge
Group Challenge Mode: Transform your simulator into a multiplayer experience by setting up a “pass-the-dice” mode. Each player takes turns rolling to see who gets the six first, and the simulator keeps track of everyone’s attempts. It’s a family game night twist that’ll have everyone on edge!
Full Source Code
Once you’ve nailed the challenge, share your updated version here in the project’s repository GitHub! Let’s grow the project together and learn as a community.
The complete source code is in the GitHub link provided above.