Now imagine there is a file📝 that you can download online. Which has a dream✨ life that you want to live and allows you to live it once. You download it and want to edit🛠 some things in that file. It is not possible to edit that file the way you edit other .txt files. But, being a Pythonista you can edit that file in python🐍.
I know that it might(ok does) sound stupid but it’s a good way to understand how to use files in python.
Are ready to edit your dream✨ world to make it even better? Let’s get started…
Contents
Previous post’s challenge’s solution
Here’s the solution to the previous post’s challenge:
print("""
%\ %\\
%% \ %% \
%% \ %% \
%%% \ %%% \
%%% \ %%% \
%%%% \ %%%% \
%%%% \%%%% \
%%%%% %%%%% \
%%%%% .-%%%%%,---------------.
.'.' | Welcome to |
.-' / | Mt. suggestive|
_.-' : `-------++------'
.--'" `. ||
,' `-._ ||
: `--..._____||_________
:
`.
""")
def suggest_places(country):
"""This functions takes country as input and returns a list of places to travel there.
If the country is not in the dictionary, then returns no idea."""
places_to_travel = {
"Hungary":["Szentendre", "Budapest", "Esztergom", "Eger"],
"Switzerland":["Matterhorn", "Jungfraujoch", "Interlaken"],
"United States":["New York", "San Francisco", "The Grand Canyon", "Houston"],
"France":["Paris", "Marseille", "Lyon"]
}
countries_available = list(places_to_travel.keys())
if country in list(countries_available):
places = "\n".join(places_to_travel[country])
return f'#>>Some places to travel in {country}:-\n{places}'
else:
return f"\nno idea.\nTry any of these countries\n{countries_available}"
print(suggest_places("Hungary"))
print(suggest_places("monkey"))
Output:
%\ %\
%% \ %% \
%% \ %% \
%%% \ %%% \
%%% \ %%% \
%%%% \ %%%% \
%%%% \%%%% \
%%%%% %%%%% \
%%%%% .-%%%%%,---------------.
.'.' | Welcome to |
.-' / | Mt. suggestive|
_.-' : `-------++------'
.--'" `. ||
,' `-._ ||
: `--..._____||_________
:
`.
#>>Some places to travel in Hungary:-
Szentendre
Budapest
Esztergom
Eger
no idea.
Try any of these countries
['Hungary', 'Switzerland', 'United States', 'France']
- First, we created a function
suggest_places
that takescountry
as a required argument. - Inside the
suggest_places
we made a nested dictionary that stores countries as its key and places to travel as values in the form of a list. - Then we used the if-else statement and the dictionary’s key function to check whether the country given is present inside the dictionary or not.
- If it was present we returned the places using the dictionary’s accessing and string’s join method in the form of a typical list.
- If not then we returned an f-string saying no idea and giving the countries available.
What is the need to use files?
I just told you in the introduction, to edit a file that has your dream life😜. Ok on a serious note, whatever you do with your program stays till the program is working, like the value of variables.
But, sometimes you might want the output generated by your program to be stored permanently. Maybe data of users signed in on your website, or players having an account in your game. The reason can be anything but it would be important to store the data permanently.
And another reason for using a file in python is that you might want to work with a file’s data in python. But you don’t want to copy that content and paste it into a string.
A string of 100 lines or more will make the program unnecessarily complicated to read, understand, use, and debug. It’s better to use the data of the file from the file only.
Opening a file
First, create a new folder on your desktop. Then open the folder in VS Code or any other text editor.
To start working with files either create a .txt file of your own in the same folder or download the text file below in the same folder to save your time.
Now go to your python file and write:-
dream = open("dream_life.txt")
print(dream)
Output:
<_io.TextIOWrapper name='dream_life.txt' mode='r' encoding='cp1252'>
This is how we open a file in python. We use the open
function for this. And assign the file object generated from it to a variable in our case dream
.
The open function takes the file path in form of a string as a required argument. And it has a default argument mode
in which we need to specify whether we want to read, write, or append a text or a binary file.
By default, it opens the file in the read text mode. You can see that in the output above too.
It has given this as output and not the content of the file because the open function only returns a file object.
We need to use read, write, and append specific functions to be able to do these operations on the dream_life.txt
.
**NOTE: you will need to mention the complete path of the file you are working with in order to access a file that is not in the same folder.**
Reading a file
Reading the complete file
To read the complete file in python we will use the read
function on the file object.
dream = open("dream_life.txt")
content = dream.read()
print(content)
Output:
I woke up today to see I am in a big beautiful valley. In here is a small cottage.
This cottage nearly has everything required for comfortable living.
And here's a little cute pet dog to play with me.
And a super-powerful custom personal computer that I designed.
Along with an ultra-thin, lightweight brand new laptop and a phone that I always dreamed of.
Above all everything here is extremely environmental friendly.
In short this cottage has everything I dreamed for.
Reading the first few characters
In case you only want to read a few characters from the start. Mention the number of characters you want to read inside the read function.
dream = open("dream_life.txt")
content = dream.read(6)
print(content)
Output:
I woke
Here’s a thing to remember. If you give the same next time you’ll get the next 6 characters
dream = open("dream_life.txt")
content = dream.read(6)
more_content = dream.read(6)
print(f"content:{content}")
print(f"more_content:{more_content}")
Output:
content:I woke
more_content: up to
**NOTE: space is also considered a character.**
Reading the first line
In case you only want to read the first line or want to read your dream life line-by-line you’ll need to use the readline
function.
dream = open("dream_life.txt")
first_line = dream.readline()
second_line = dream.readline()
print(f"first_line:{first_line}", end="")
print(f"second_line:{second_line}")
Output:
first_line:I woke up today to see I am in a big beautiful valley. In here is a small cottage.
second_line:This cottage nearly has everything required for comfortable living.
**NOTE: Inside the print function we have given end=""
to prevent extra lines due to the print function.**
Reading in the form of a list
In case you want the lines of the file in the form of a list you can use the readlines
function
dream = open("dream_life.txt")
line_list = dream.readlines()
print(line_list)
Output:
['I woke up today to see I am in a big beautiful valley. In here is a small
cottage.\n', 'This cottage nearly has everything required for comfortable
living.\n', "And here's a little cute pet dog to play with me.\n", 'And a
super-powerful custom personal computer that I designed.\n', 'Along with
an ultra-thin, lightweight brand new laptop and a phone that I always
dreamed of.\n', 'Above all everything here is extremely
environmental friendly. \n', 'In short this cottage has everything I
dreamed for.\n']
Writing/Creating
Now that we have read what we had in our dream_life
it’s time to make some changes to that because there’s a big chance that you did not like it the way it is. (Afterall, I have written it, So let’s see the possible customization)
“w” Mode
This mode will overwrite the content inside dream_life
. And if the dream_life
doesn’t exist will create a new dream_life
(file obviously).
So, you can use this if you kind of hate or don’t like it much enough the dream_life
that I have created.
To write inside dream_life
you need to first open it in write mode. Then use the write function to write the content you want to.
dream = open("dream_life.txt", "w")
dream.write("""Write here the dream life you wish to have.\n
This will overwrite the original file.\n
Consider Copying the already existing file's content.""")
This time there would be no output displayed instead the content
inside file will change to the one you gave inside the write function.
#Content inside dream_life.txt:-
Write here the dream life you wish to have.
This will overwrite the original file.
Consider Copying the already existing file's content.
**NOTE: \n
is to store the content inside the file in a new line.**
Till now we have just talked about the “mode” attribute inside the open
function. This time we have used it to define that we want to open dream_life
in writing mode.
(reading is the default mode. You can also explicitly mention reading mode by writing "r"
instead of "w"
)
“a” Mode
In case you think that you don’t want to completely change the content inside the dream_life
then you can use append mode. This will simply add the content at last in dream_life
. And if the dream_life doesn’t exist will create a new dream_life
(file obviously).
To append the content in dream_life
you need to open it in the append mode. Then use the write
function to write the content you want to add to it.
dream = open("dream_life.txt", "a")
dream.write("Write here the lines you want to add to the dream_life.")
This time too there would be no output displayed instead the content you gave
inside the write function will be added to the file.
#Content inside dream_life.txt:-
I woke up today to see I am in a big beautiful valley. In here is a small cottage.
This cottage nearly has everything required for comfortable living.
And here's a little cute pet dog to play with me.
And a super-powerful custom personal computer that I designed.
Along with an ultra-thin, lightweight brand new laptop and a phone that I always dreamed of.
Above all everything here is extremely environmental friendly.
In short this cottage has everything I dreamed for.
Write here the lines you want to add to the dream_life.
“x” Mode
There’s a possibility that you don’t know what you’ll write inside dream_life
if you completely change it and also have no idea what will you add to it and you like the original one a bit.
You should this time open the file in "x"
mode. It will simply create a new file with the content you wrote inside the write function.
**NOTE: It will produce an error if the file already exists so try to give it another name.**
dream = open("dream_life1.txt", "x")
dream.write("""Write your own version of dream_life.\n
It will be stored in a new file.\n
If the file already exists, it will give an error""")
This time too there would be no output displayed instead a new file will be
created having your version of dream life.
#Content inside dream_life1.txt:-
Write your own version of dream_life.
It will be stored in a new file.
If the file already exists, it will give an error
Closing a file
It is super important to close a file after you are done working with it. If you don’t follow this practice, sooner or later you’ll encounter unexpected errors, issues, and outputs.
To close a file you just need to write the:-
variable_which_stores_it.close()
#in our case
dream.close()
I suggest closing the file as soon as you open it. And then in between the open and close do perform the operations. Example:-
dream = open("dream_life.txt", "x")
#code the operations here
dream.close()
“with open” block for opening file
Now if you think you’ll forget to close the file then you should use with open
block to open a file. If you open a file this way then there would be no need to close it. Here’s how you can open a file using a with open
block:-
with open("dream_life.txt", "r") as dream:
first_line = dream.readline()
print(first_line)
Output:
I woke up today to see I am in a big beautiful valley. In here is a small cottage.
To use this you first need to write the with
and the open
keyword.
Then inside a parenthesis give the file name and mode you want it to open in just as we did before.
After that use the keyword as
to store the file object inside a variable.
Finally, give the variable name and a colon.
Then you can write all the operations you want to do inside this block.
Image file
Remember when we opened the file I said that it by default opens in the read text mode. Then we also saw how we can open it in write text mode, append text mode, and “x” text mode.
But, I did also mention that files can be opened in binary. One use of the binary mode is on image files and we are gonna see that here.
For this, you should first have an image. Select one from your computer and bring it to the folder you are in. Or you can download the image below to the folder directly.
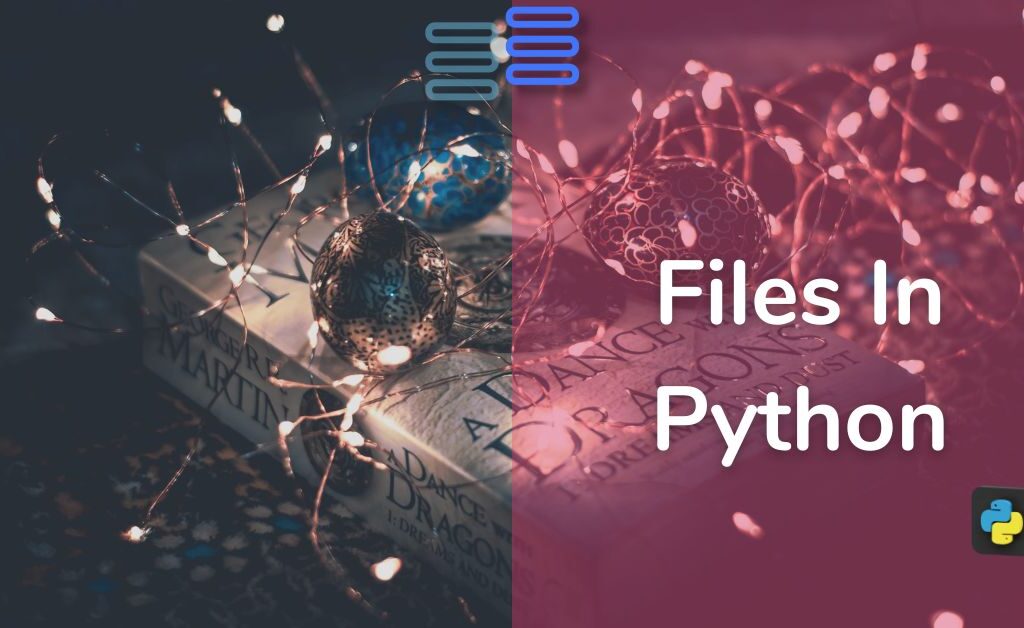
Open
To open an image file write the way you used to open a normal .txt file. This time you need to add a "b"
in the mode attribute like:-
#in read mode
with open("File Handling In Python Your Dream Your Way.jpg", "rb") as image:
#in write mode
with open("File Handling In Python Your Dream Your Way.jpg", "wb") as image:
Read
To read an image file you’ll need to open it in the read binary mode.
#in read mode
with open("File Handling In Python Your Dream Your Way.jpg", "rb") as image:
print(image.read())
When you run this you’ll see a bunch of text being printed as output. This is the binary text of the image. And that’s how you read an image file.
Write
To learn to write in the image file we will create a copy of it.
For this, we first need to read the image file and store its content in a variable.
Then we would need to create a new image file using the wb
mode of the open function.
Lastly, we would need to write the content of the variable inside a new image.
image = open("File Handling In Python Your Dream Your Way.jpg", "rb")
#copying the content of this image file in binary form.
content = image.read()
image.close()
image2 = open("Copy_image.jpg", "wb")
#writing the copied file.
image2.write(content)
No output will be shown when you run this program however a new image file with name Copy_image.jpg will be created.
Delete a file
Let’s say you are loving your version of the dream_life
and no longer need the one that you downloaded.
So to delete the file we would need to import a module, os
. It is a module that helps us work with files, directories, and the operating system.
We will use the remove function of the os
module. To import the module type:
import os
Then to use its function type:
os.remove("dream_life.txt")
Now, as soon as you run this program the dream_life
will be deleted.
Rename a file
Maybe you are extremely serious🤨 about what you have written in your dream_life
file and these are serious goals of your life.
So, you might want to rename the file as goals_in_life
📝.
The os module has a function for that.👍
os.rename("dream_life.txt", "goals_in_life.txt")
Run the program and the file will be renamed if exists. ✔
If the dream_life
does not exist FileNotFoundError
❗ will be raised.
And if goals_in_life
already exists then FileExistsError
❗ will be raised.
Here’s a reference to the python file’s method which has these methods/functions and a lot more. Click here to go there.
Conclusion
Ok so in this post we first downloaded a file that cannot be edited normally and contains your dream life(just an assumption).
Then we found multiple ways to read and store its content in python.
Next, we saw ways to edit it.
After that, we saw how we can work with images in python.
And in between we covered topics like opening, closing, deleting a file, renaming a file, and then the with open block to open files.
Challenge🧗♀️
Your challenge is to create a game rock, paper, and scissors game. And store data of wins and losses of player1 and player2 in the results file. Remember the game should be a 2 player game.
You might need to use:
import os
#This function clears the screen/console in python.
os.system('cls')
The result.txt should look like this:-
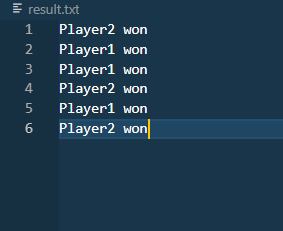
Go fast. I am waiting. Comment your answers below.
This was it for the post. Comment below suggestions and tell me whether you liked it or not. Do consider sharing this with your friends.
See you in the next post till then have a great time.😊