Do errors irritate you?
These usually occur due to two main reasons
- wrong syntax(like forgetting colons, indentation, or parenthesis.);
- exceptions like TypeError(when you try to add a string with an integer.), ZeroDivisionError(when you try to divide a number with zero.)
Exceptions occur due to three reasons:
- incorrect logic;
- invalid input by the user; or
- some process that is likely to result in errors.
With practice, you won’t suffer from the problem of logic or syntax. But it is hard to predict all the possible invalid inputs and solve the problem of error-prone procedures.
But then how will you write robust 💪software that works in all situations?
In this post, we’ll see try and except statements with another two blocks, which will help you solve this problem.
By taking an example of four friends who want a bar of chocolate.
I know that it sounds a bit crazy but I think it’s a good way to visualize errors.
Contents
Previous post’s challenge’s solution
Here’s the solution to the previous post’s challenge:
- First, you need to import the time module and need to print the instructions for using the stopwatch.
- Then you need to start counting the time when the user presses enter. For this, we took input from the user and after that stored the
current_time
using thetime
function of the time module. - Next, we created an empty list to store all the laps taken during a go.
- After that, we gave a try and except statement.
The python interpreter tries to execute the code inside the try block and if the code produces the given exception/error then it executes the except block.
We would need to stop the time of the stopwatch whenever ctrl c
is pressed. When you press ctrl c
python considers it as a KeyboardInterrupt
error.
That’s why we used the try and except in our program. This would help us take laps every time we press enter. And will help us stop the time when we press ctrl c
.
import time
print("\nEnter to begin. Then press enter everytime you want a lap. Finally press ctrl+c to stop tracking the time.", end="")
start = input()
print("started counting\n")
current_time = time.time()
lap_time = []
try:
while True:
lap = input()
lapTime = round(time.time(), 2)
lap_time.append(lapTime)
print(f"Lap# {len(lap_time)} = {round(lapTime - current_time, 2)}", end ="")
except KeyboardInterrupt:
print("\n\nDone")
stop_time = time.time()
difference = round(stop_time - current_time, 2)
print(f"Total time difference = {difference}")
Output:
Enter to begin. Then press enter everytime you want a lap. Finally press ctrl+c to stop tracking the time.
started counting
Lap# 1 = 1.4
Lap# 2 = 2.11
Lap# 3 = 3.5
Lap# 4 = 4.78
Lap# 5 = 6.43
Lap# 6 = 7.63
Done
Total time difference = 9.76
In any case, if you feel unable to understand the program you should have a problem with any of these: import, while loop, lists, variables, strings, f-strings, or escape sequences.
If you know the concepts above well then you should consider reading this post well because the confusion may be because of try and except.
Exceptions
Built-in
The four friends Luke, Harry, Mason, and Michelle got a map to reach the tastiest🤤 chocolate🍫 in the world.
Now, they want to calculate how much money💵 they have so that they could buy the chocolate🍫🧗♀️ when they reach there.
So they wrote this code:
luke = 10
harry = "20"
mason = 25
michelle = 45
print(f"Total amount of money = {luke+harry+mason+michelle}")
Output:
Traceback (most recent call last):
File "c:\python-hub.com\try_except_exceptions.py", line 6, in <module>
print(f"Total amount of money = {luke+harry+mason+michelle}")
TypeError: unsupported operand type(s) for +: 'int' and 'str'
It produced an error because harry
gave 20
inside of quotations and python considered it a string. And as you know that we can not add a string with a number.
These errors, that are detected during execution are called exceptions.
Even if a statement or expression is syntactically correct, it may cause an error when an attempt is made to execute it. Like it happened in the above case.
There are a number of exceptions in python:-
Exception | Cause |
---|---|
IndexError | Raised when the index of a sequence is out of range. |
KeyError | Raised when a key isn’t found in a dictionary. |
KeyboardInterrupt | Raised when we press ctrl c or delete . |
NameError | Raised when a variable is not found. |
RuntimeError | Raised when the error doesn’t fall under any other category. |
TypeError | Raised when a function/operation is applied to an incorrect data type. |
ZeroDivisionError | Raised when a number is divided by 0 . |
ValueError | Raised when an operation or function receives an argument that has the right type but an inappropriate value |
To read about all the possible built-in exceptions click here. To see the hierarchy of exceptions click here.
Handling exceptions with try and except
So all four friends🤘 have decided to make a copy for each of them of the image of the map📜 that they have got.
For this, they decided to open the image file in python and create four copies of it and keep the original image safe🗝🔑.
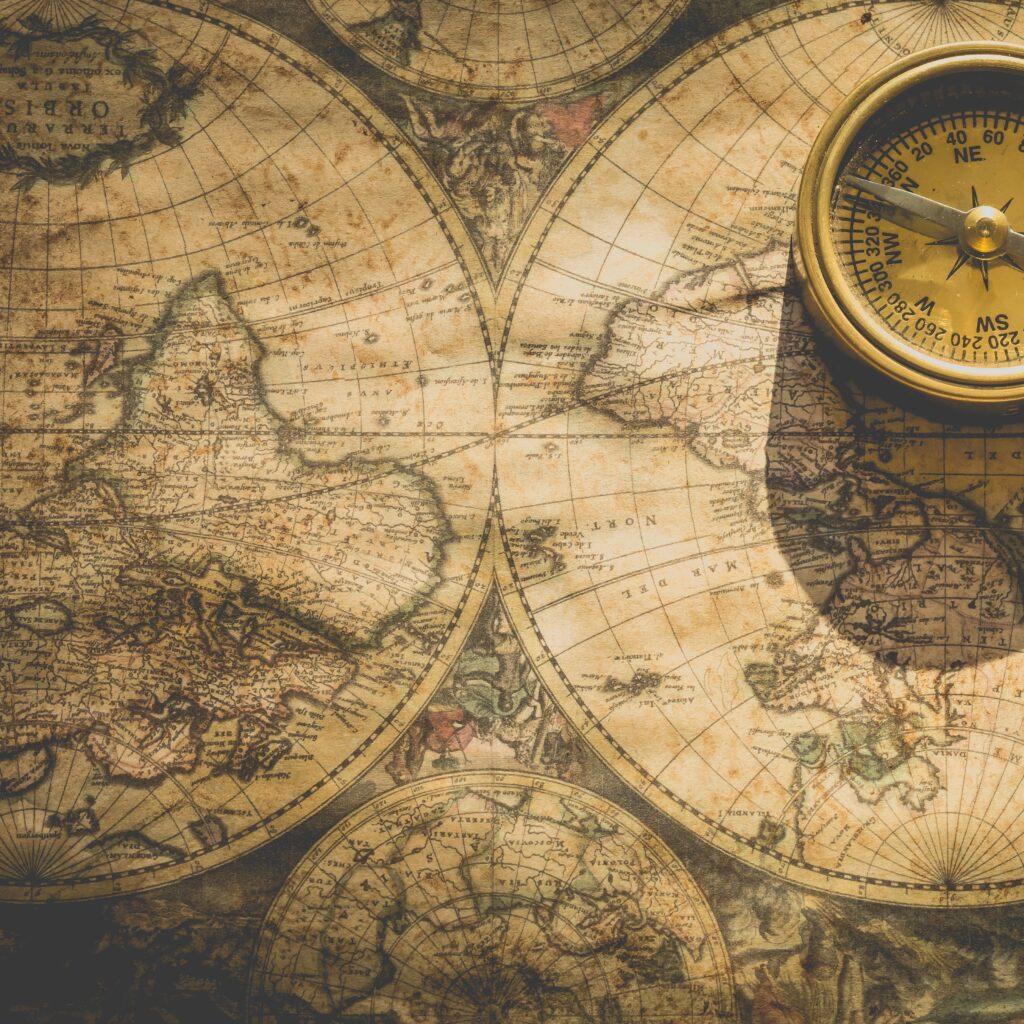
Here’s the code that they wrote:
with open("map for chocolat.jpg", "rb") as map:
map_content = map.read()
for i in range(1, 5):
with open(f"map for chocolate{1}.jpg", "wb") as map:
map.write(map_content)
Output:
Traceback (most recent call last):
File "c:\python-hub.com\try_except_exceptions.py", line 1, in <module>
with open("map for chocolat.jpg", "rb") as map:
FileNotFoundError: [Errno 2] No such file or directory: 'map for chocolat.jpg'
Oh no, error❌ once again. This error(ok exception) arose because they wrote the filename wrong. Look how they missed an e
at the end of the image name.
Is there a way to tell this to them more clearly and gently? Yes, using a try and except. Let’s see how.
try:
with open("map for chocolat.jpg", "rb") as map:
map_content = map.read()
for i in range(1, 5):
with open(f"map for chocolate{1}.jpg", "wb") as map:
map.write(map_content)
except Exception as e:
print(e)
Output:
[Errno 2] No such file or directory: 'map for chocolat.jpg'
See how clearly the problem is expressed this time no tracebacks and file names.
Here’s what we did:
- First, we told python to try to open the image file and then paste it to the 4 files.
- We told python if it fails to do so it should except that something has gone wrong.
- Then we stored what is gone wrong in the variable e.
- Thus, we excepted our Exception and stored it to e variable, and then printed it to let the user know what’s gone wrong.
You can write any code below except. It may be a custom print statement describing the possible error. Or can be a code block that you want to execute.
Specific exceptions
You can accept specific exceptions if you somehow have an idea💡 of what can go wrong❌.
Like say for example you already have an idea💡 that any of the 4 would enter a string instead of an integer then you can give a ValueError❗:
try:
luke = int(input("Luke: "))
harry = int(input("Harry: "))
mason = int(input("Mason: "))
michelle = int(input("Michelle: "))
except ValueError:
print("One of you entered a string!!")
Output:
Luke: 10
Harry: 20
Mason: twenty five
One of you entered a string!!
Here we excepted a specific exception. In case of any other error, it won’t work.
**And yes you can give any number of except after try. But, can never give a try without any except.**
The else block
All four of them decided to compare the distances of the two roads that would lead them to the chocolate.
For this, they want to print which among the following is longer.
Now you can consider writing it with a simple try and except statement because you know that these kids do usually enter strings instead of numbers.
But I think using an else along with try and except would be a better idea:
try:
distance1 = 10
distance2 = 20
compare = distance1 > distance2
except ValueError:
print("You entered a string!!")
else:
if compare == True:
print("distance1 is longer.")
elif compare == False:
print("distance2 is longer.")
Output:
distance2 is longer.
**The else block is always written below the except block(s).**
❗This block is only executed if the try block is executed without any errors.
In our case, we wanted to see which of the two distances is longer.
Luckily the kids gave both values in the form of an integer this time.
As a result of which the comparison inside of the try block was executed successfully.
And that’s why the else block was executed telling which among them was longer.
The finally block: To clean up
The kids now decided to write all they know about the place in a file. They just want to transfer the information they have stored in variables about that place to the file.
Here in this case there is a strong chance that some error occurs while working with the file and in that case, the file won’t be closed after you’ve opened it.
And you know that it is not in any way good to leave a file open after working with it. The finally
block is gonna be helpful in this case:
luke = "we have enough money to but it."
harry = "we have 4 copies of the map."
michelle = "We have figured the shortest route to there."
try:
place_info = open("place_information.txt", "w")
place_info.write(
f"""Luke: '{luke}'\nHarry: '{harry}'\nMichelle: {michelle}""")
print("Execution successful.")
except:
print("OOPs something went wrong!")
finally: #This will be executed no matter what.
print("finally was executed.")
place_info.close()
Output:
Execution successful.
finally was executed.
In this case, there was no error, and the code was executed smoothly after that the finally
block was executed.
This block allows you to execute code no matter what is the result of try and except.
For our case, it closed the file.
The raise keyword
Are you excited to know whether the kids got the chocolate or not and what was so special about the chocolate?
Well, because these kids:
- studied the map🧐 carefully✅,
- figured out the shortest route✅,
- and counted the money💵 which turned out to be enough to buy that chocolate✅.
They were able to reach the place where they were to buy the chocolate.
When they reached there they saw a big chocolate city. Then an angel👼 arrived and give them a program which was to test whether they deserve the chocolate or not:
exercise_daily = 3
eat_healthy_daily = "sometimes"
drink_enough_water_daily = "no"
brush_teeth_daily = "yes"
qualify = [exercise_daily, eat_healthy_daily, drink_enough_water_daily, brush_teeth_daily]
for i in qualify:
if type(i) is int:
raise TypeError("Numbers aren't allowed.")
elif i.startswith("y"):
print("Passed")
elif i.startswith("n"):
print("Failed!!")
else:
print("Just answer in yes or no!")
Output:
Traceback (most recent call last):
File "c:\python-hub.com\try_except_exceptions.py", line 10, in <module>
raise TypeError("Numbers aren't allowed.")
TypeError: Numbers aren't allowed.
Notice they have used a keyword raise
in the program.
The program they wrote will break and raise an error if, in any of the given variables, the kids entered a number.
That’s the use of the raise
keyword. While the try and except help to handle exceptions, the raise
helps to raise them.
This is useful because we can save the program’s running time and prevent the program from storing the wrong input.
Imagine what if the code below this possible exception was of 1000 lines. And you would have to go through each one of them to know if the input was incorrect. This would have saved you a lot of time⏲ and memory space.
Did they get the chocolate?
And the most awaited🤩(maybe I am exaggerating) answer is yes they did get the chocolate🍫. They adopted all these good habits👍 and got it. Do you know what was special✨ about it? It was made of everything that is good🥗 for health, a complete nutritious diet.
The angel👼 told them that they shouldn’t ever eat any refined sugars as it is extremely harmful❌ to their health. And that they should follow these good habits all their life to become happy forever and not for a few minutes while they eat chocolate🍫.
They got a bar of dark chocolate🍫 each as a bonus for following these good habits.
Conclusion
We saw that the
- kids counted how much money they got,
- created a copy of the map for each one of them,
- figured out the shortest distance to the place,
- wrote a clear description to reach the place,
- and finally went there and made themselves deserving of having the chocolate.
And, good news🤩😋, got it too.
Now, this post does not cover how to define your own exceptions we will take a look at them when we will be going through OOP concepts.
Try and except official documentation click here.
Challenge
Now that you know what is try, except, else, and finally in python, your challenge is to create a flow chart showing the flow of the program when you use all of these in your program.
Go fast. I am waiting. Comment your answers below.
This was it for the post. Comment below suggestions if there and tell me whether you liked it or not. Do consider sharing this with your friends.
See you in the next post till then have a great time.😊