Efficiently Update Firebase Firestore Data in Flutter Using BLoC Pattern
Editing your profile should feel simple and seamless, right?
That’s why today, we’re taking the first step toward making profiles fully editable through the UI—directly updating Firestore using Flutter & BLoC.
Here’s what we’re doing:
✅ Fetching user profiles dynamically
✅ Passing data correctly across mobile & desktop layouts
✅ Designing an Edit Profile page
✅ Adding functionality to modify the user’s bio in Firestore
By the end of this, you’ll be able to update user details straight from the app, making Connect feel more real and interactive.
Let’s dive in.
Github Repo: https://github.com/maitry4/Connect/
All Articles Sequentially: https://python-hub.com/category/connect-social-app/
Efficiently Update Firebase Firestore Data in Flutter Using BLoC Pattern Efficiently Update Firebase Firestore Data in Flutter Using BLoC Pattern
Contents
Efficiently Update Firebase Firestore Data in Flutter Using BLoC Pattern Efficiently Update Firebase Firestore Data in Flutter Using BLoC Pattern Efficiently Update Firebase Firestore Data in Flutter Using BLoC Pattern
Step 1: Prepare The Profile Page
The profile page wouldn’t display the information of the current user only. Why?
Well, because I’ll visit other’s profiles as well. Right?
That’s why let’s first start by providing the profile page with the user’s id.
The information there would be displayed based on the uid only and not the current user.
In the main_screen.dart
, create the list of pages inside the _CMainScreenState
.
Above this get the user ID.
class _CMainScreenState extends State<CMainScreen> {
final SidebarXController _controller = SidebarXController(selectedIndex: 0);
@override
Widget build(BuildContext context) {
final user = context.read<CAuthCubit>().currentUser;
final uid = user!.uid;
final List<Widget> pages = [
const CHomePage(),
CProfilePage(uid: uid),
const CSettingsPage(),
];
Load the user’s profile data in the init
method using the profile cubit.
// cubits
late final authCubit = context.read<CAuthCubit>();
late final profileCubit = context.read<CProfileCubit>();
// get the current user
late CAppUser? currentUser = authCubit.currentUser;
// onStartup
@override
void initState() {
super.initState();
// load the user profile data.
profileCubit.fetchUserProfile(widget.uid);
}
Test if everything works fine.
Now, inside the profile page, we will use a Bloc Builder instead of the Scaffold.
There can be 3 states:
- Loaded: Here we can show our Scaffold as it is.
- Loading: Here we will call our loading screen.
- Error: Here we will simply show “Profile Not Found… “
➡️Click To View the Code
return BlocBuilder<CProfileCubit, CProfileState>(
builder: (context, state) {
// loaded
if (state is CProfileLoadedState) {
// get the current user
late CProfileUser? profileUser = state.profileUser;
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.surface,
),
backgroundColor: Theme.of(context).colorScheme.surface,
body: Center(
child: SafeArea(
child: isDesktop
? _buildDesktopLayout(context, res, profileUser)
: _buildMobileLayout(context, res, profileUser),
),
),
);
}
// loading
else if (state is CProfileLoadingState) {
return const CLoadingScreen(loadingText: "Loading Your Profile...",);
}
else {
return const Center(child: Text("Profile Not Found"));
}
});
}
You can remove the auth cubit completely now
Test if everything works fine.
Efficiently Update Firebase Firestore Data in Flutter Using BLoC Pattern Efficiently Update Firebase Firestore Data in Flutter Using BLoC Pattern Efficiently Update Firebase Firestore Data in Flutter Using BLoC Pattern
Step 2: Add A ProfileCubit To The app.dart And Fetch User Data In The Profile Page
Add this in the app.dart
// repos
final authRepo = CFirebaseAuthRepo();
final profileRepo = CFirebaseProfileRepo();
...
// profile cubit
BlocProvider(
create: (context) => CProfileCubit(profileRepo: profileRepo),
),
In the mobile layout build method and the desktop layout build method go ahead and add another argument for the profile user.
Pass the profile user to the profile card widget and fetch the name and bio from there. You can go to Firebase and add a dummy bio therein.
➡️Click To View Profile Page Modifications
Widget _buildDesktopLayout(BuildContext context, ResponsiveHelper res, CProfileUser usr) {
return Center(child: CProfileCardWidget(user: usr));
}
Widget _buildMobileLayout(BuildContext context, ResponsiveHelper res, CProfileUser usr) {
//mobile
Text(
usr.name,
style: const TextStyle(fontSize: 20, fontWeight: FontWeight.bold),
),
SizedBox(width:res.width(50),child: Text(usr.bio, textAlign: TextAlign.center, style: TextStyle(fontSize: 14))),
➡️Click To View The Profile Card Widget Modifications.
class CProfileCardWidget extends StatefulWidget {
final CProfileUser user;
const CProfileCardWidget({super.key, required this.user});
...
Text(widget.user.name, style: TextStyle(color:Theme.of(context).colorScheme.tertiary, fontSize: 28, fontWeight: FontWeight.bold)),
const SizedBox(height: 10),
SizedBox(width:res.width(20),child: Text(widget.user.bio, textAlign: TextAlign.center, style: const TextStyle(fontSize: 14))),
Efficiently Update Firebase Firestore Data in Flutter Using BLoC Pattern Efficiently Update Firebase Firestore Data in Flutter Using BLoC Pattern Efficiently Update Firebase Firestore Data in Flutter Using BLoC Pattern
Step 3: Design An Edit Profile Page
The code is pretty long that’s why I am just providing you with a brief explanation.
The code can be found on Github Repo: https://github.com/maitry4/Connect/
Major Components of the Profile Edit Page:
- Profile Image Picker (
CProfileImagePicker
) – Displays a circular avatar with an option to pick a new profile image. - Bio Input Field (
CBioInputField
) – A text field allowing users to update their bio with an icon for editing. - Action Buttons (
CActionButton
) – Two buttons: “Save” (to confirm changes) and “Cancel” (to discard changes and go back). - Responsive Design (
ResponsiveHelper
) – Ensures all UI elements scale properly across different screen sizes. - AppBar – Displays the title “Edit Profile” with a back button for navigation.
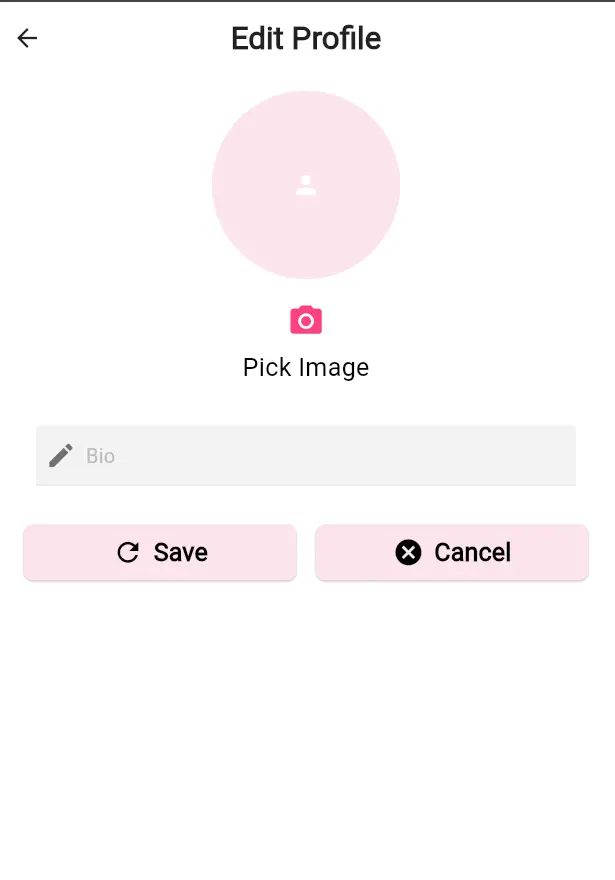
Step 4: Make The Edit Profile Page Responsive
To make the edit page responsive. We’ll first create a profile edit page design for desktop.
In features/profile/presentation/components/
create profile_edit_desktop.dart
.
After this make two methods in the profile edit page, one for mobile where we will keep the existing code.
And in another return the profile_edit_desktop.dart
.
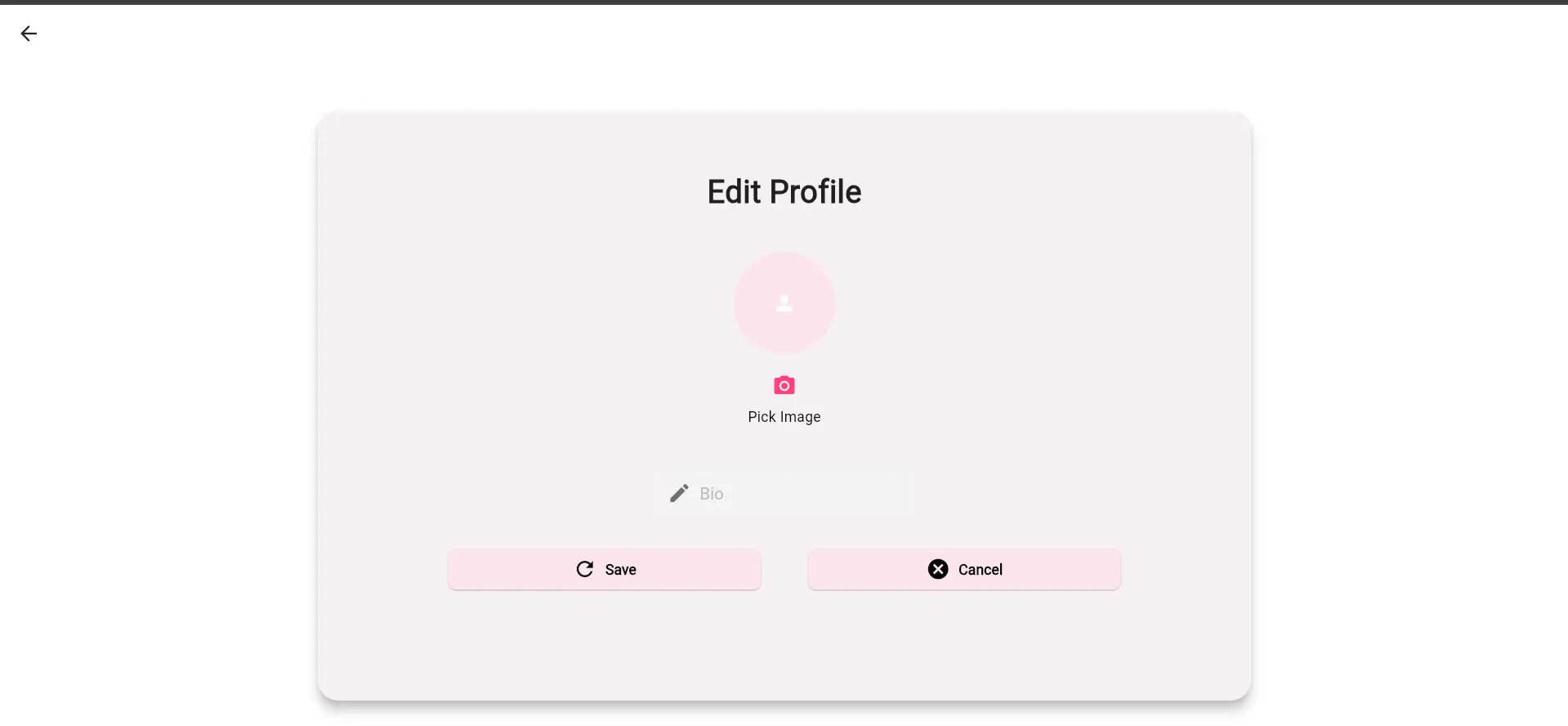
That’s it you can view these codes in the GitHub Repo provided.
Step 5: Add Functionality To Edit The Bio
Move the Scaffold to a new buildEditPage
method.
And in the main build method go ahead and return a BlocConsumer.
There are 2 possibilities here:
- Profile data is still loading
- Everything is successfully loaded
For the last case we will return our buildEditPage
method.
Widget build(BuildContext context) {
return BlocConsumer<CProfileCubit, CProfileState>(
builder: (context, state) {
// profile loading
//edit form
return buildEditPage();
},
listener: (context, state) {
},);
}
Widget buildEditPage({double uploadProgress = 0.0}) ...
Now return the loading screen if the state is loading.
And in the listener if the state is loaded return the profile page.
Test if you are able to update the bio.
That’s it for today.
Conclusion
In the next article, we will see how to work with the Profile Image of the user using the Firebase storage.
With today’s progress, the foundation for dynamic user profiles is set.
Now, users can fetch and edit their bio directly from the UI.
Tomorrow, we’re stepping it up a notch—working with profile images using Firebase Storage.
Stay tuned! 🚀