Hello Pythonistas, welcome back. As you know by now there are 6 types of operators in Python. We discussed the first 3 in the previous article.
In this, we are going to take a look at the other 3 and Operator precedence.
So let’s dive straight into it.
Contents
Previous post’s challenge’s solution
Check whether your answer is correct or not. Solution link.
Operators in python
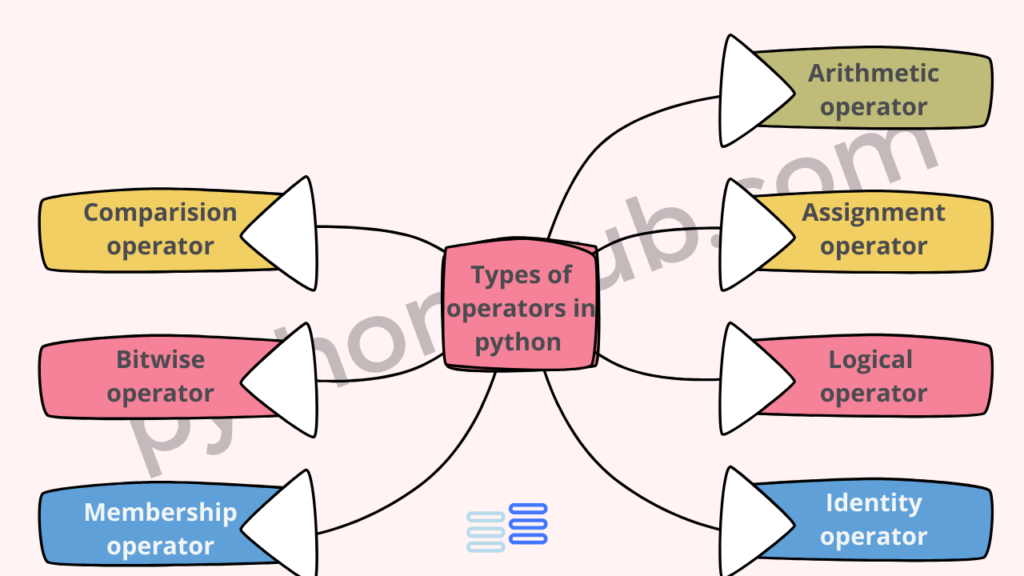
Logical
Well, have you ever been told by anyone to think logically🤔 using your brain🧠?
I had to listen to it a hell lot of times.
Now, I am not sure whether I think logically🤔 or stupidly😅 using my brain🧠. But, python has got tools to make your program think🧠 logically. Here are those three tools:
Operator | Function | Example |
---|---|---|
and | This returns True if both the statements are true | print(10==10 and 10!=11) #True |
or | This returns True if at least one of the statements is true | print(10!=10 or 10!=11) #True |
not | If the result is True it will give False | print(not(10==10)) #False |
Note: If you are unable to understand what returns mean it is the result we get on using a function or operators that can be stored inside a variable or be printed using the print
function. Don’t worry if you still feel confused it would be discussed in detail when I will explain the functions.
Identity
Whenever you create a variable in python it points out a location in memory because python is dynamically typed.
These operators check whether two variables or even values point to the same memory location or not.
Operator | Function | Example |
---|---|---|
is | Returns True if both point at the same location in memory | a=10 |
is not | Returns False if both point at the same location in memory | a=10 |
Membership
Have you ever joined a club, a football or basketball club?
If yes, how do you check whether you are a member?
Maybe by using a computer database or looking into the list of members.
Python uses membership operators to check if the value you entered is present in the sequence(say, list. It is a datatype) or not.
Operator | Function | Example |
---|---|---|
in | Returns True if the specified value is present in the given sequence. | L = [1,2,3,10] |
not in | Returns False if the specified value is present in the given sequence. | L = [1,2,3,10] |
Operator Precedence
Ok, now that you know all the different types of operators in python, and what are they used for it’s time to look at their precedence.
Don’t be scared by the word it just means priority.
To be more specific which operator will be given priority when two or more are used.
An example will make this crystal clear.
print(10 - 4 * 2) #What do you think it will print? 12 or 2
It will print 2
because *
has higher precedence compared to -
.
I hope you got the point now. So the operator precedence in python is:-
- In the United States, we use an acronym called PEMDAS which stands for:
- Parentheses Exponents Multiplication Division Addition Subtraction.
- That’s the order Python follows as well.
But it is not a strict😑 order as in “Do P, then E, then M, then D, then A, and then S”.
Multiplication and division are given the same priority=).
Similarly, addition and subtraction are given the same priority=).
And among them left to right order is followed➡.
Meaning if the + sign comes before the – sign then it will be a priority. Example:
print(10+2-3) #9
Internally, python will first add 10
and 2
and then, will subtract 3
from 12
.
Operator precedence
Operator |
---|
() |
** |
* , / , // , % |
+ , - |
== , != , > , >= , < , <= ,is , is not , in , not in |
not |
and |
or |
Conclusion
Operators in python are tools🛠 that help you with logical🧠 and mathematical🔢 functions in python.
There are mainly 6 types of operators in python:- arithmetic, assignment, identity, membership, comparison, and logic.
Python doesn’t give equal importance and priority to all operators that’s why we discussed operator precedence👑🏆.
Challenge🧗♀️
Now, the challenge for this post is you have to tell the output of the following code:-
print(1+1*(4-12))
No cheating allowed use pep and paper! It’s a test of your operator and its precedence’s understanding.
Go fast. I am waiting. Comment your answers below.
This was it for the post. Comment below suggestions and tell us whether you liked it or not. Do consider sharing this with your friends.
See you in the next post till then have a great time.😊
answer: -7
Yes Khushi the correct answer indeed is -7.
You can check the explanation here