This won’t be a post with some strangest 😨🤪 examples or story-like stuff just because it is very simple💁♀️ to work with CSV files.
There are two ✌ significant ways to work with it:
- Using the CSV module
- Using the pandas library (DataFrame data structure)
But yes the post will have a fun 😄 explanation using some visuals 🤩 to make it even more simple.
You need to clear 6 🖐☝ checkpoints to learn and understand the working well. To reach🎯 each checkpoint you would need to clear certain levels🎮. Just 2 checkpoints have more than 1 level. And it’s all super easy.
Without a due let’s get started…
Contents
Before we dive into CSV let’s take a look👀 at the previous post’s challenge’s solution.
Previous post’s challenge’s solution
The whole code has comments to explain what the code is doing. Read it carefully🧐 to understand. If you still have any doubts feel free to ask in the comment section below.👍😊
Don’t forget to solve it on your own after reading it.
# strings for representing rock paper and scissors.
rock = '''
_______
---' ____)
(_____)
(_____)
(____)
---.__(___)
'''
paper = '''
_______
---' ____)____
______)
_______)
_______)
---.__________)
'''
scissors = '''
_______
---' ____)____
______)
__________)
(____)
---.__(___)
'''
# for clearing the console
import os
# all the three strings in a list
choices = [rock, paper, scissors]
# asking player1's choice from the three
player1_choice = int(input("What do you choose? Type 0 for Rock, 1 for Paper or 2 for Scissors.\n"))
# clearing the screen so that player2 cannot know player1's choice.
os.system("cls")
# asking player2's choice from the three
player2_choice = int(input("What do you choose? Type 0 for Rock, 1 for Paper or 2 for Scissors.\n"))
os.system("cls")
# COMPARING THE CHOICES OF BOTH THE PLAYERS
# If both had same choices than it would be a tie.
if player1_choice==player2_choice:
print(f"Player1 choice:\n{choices[player1_choice]}\nPlayer2 choice:\n{choices[player2_choice]}")
won = "Tie"
print("Tie")
# if player1 choose rock and player 2 choose paper. Player2 wins
elif player1_choice==0 and player2_choice==1:
print(f"Player1 choice:\n{choices[player1_choice]}\nPlayer2 choice:\n{choices[player2_choice]}")
won = "Player2 won"
print("Player2 won")
# if player1 choose paper and player 2 choose rock. Player1 wins
elif player1_choice==1 and player2_choice==0:
print(f"Player1 choice:\n{choices[player1_choice]}\nPlayer2 choice:\n{choices[player2_choice]}")
won = "Player1 won"
print("Player1 won")
# if player1 choose rock and player 2 choose rock. Player1 wins
elif player1_choice==0 and player2_choice==2:
print(f"Player1 choice:\n{choices[player1_choice]}\nPlayer2 choice:\n{choices[player2_choice]}")
won = "Player1 won"
print("Player1 won")
# if player1 choose scissors and player 2 choose rock. Player2 wins
elif player1_choice==2 and player2_choice==0:
print(f"Player1 choice:\n{choices[player1_choice]}\nPlayer2 choice:\n{choices[player2_choice]}")
won = "Player2 won"
print("Player2 won")
# if player1 choose paper and player 2 choose scissors. Player2 wins
elif player1_choice==1 and player2_choice==2:
print(f"Player1 choice:\n{choices[player1_choice]}\nPlayer2 choice:\n{choices[player2_choice]}")
won = "Player2 won"
print("Player2 won")
# if player1 choose scissors and player 2 choose paper. Player1 wins
elif player1_choice==2 and player2_choice==1:
print(f"Player1 choice:\n{choices[player1_choice]}\nPlayer2 choice:\n{choices[player2_choice]}")
won = "Player1 won"
print("Player1 won")
#Incase user gives invalid input
else:
print("invalid choice")
#Storing data in the file
with open("C:\\Users\\maitr\\Desktop\\folders\\100 Python Exercises\projects\\result.txt", "a") as result:
result.write(f"{won}\n")
#To store data in new lines
Results after playing three times in results.txt:
Player2 won
Player1 won
Tie
Go to your code editor solve it on your own and then come back. No cheatings.🤨
What are CSV files?
It stands🧍♂️ for Comma Separated Values.
CSV is a simple text file you can open on various programs, even💁♀️ on notepad.
It separates information using commas ','
. However, It can use different 🤹♀️separators(delimiters) as well.
It is useful for the exchange of structured📗 data from one program➡to another.
Here’s how a CSV file looks:
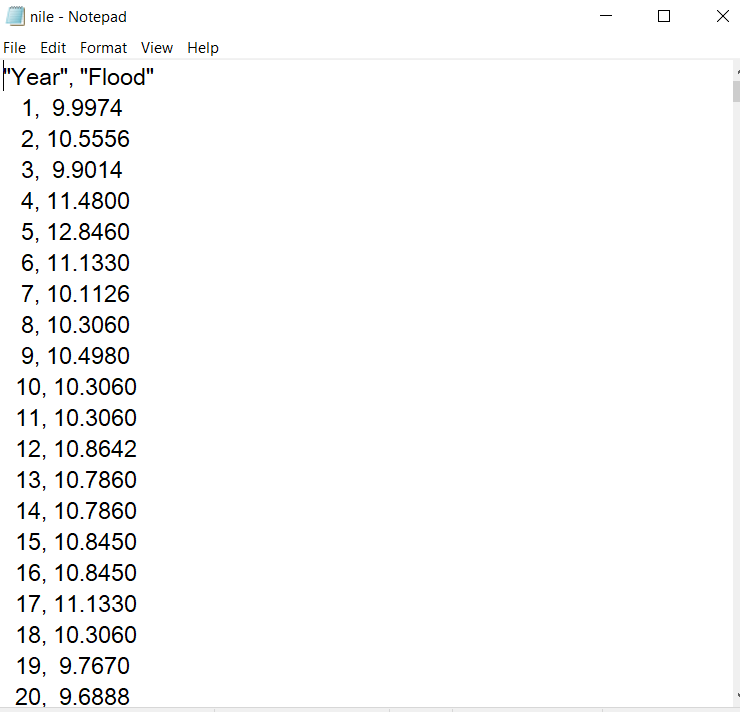
I found a good site that has a lot of sample CSV files. Click here to visit.
To dive into detail about CSV you can read this article its good too. click here.
Why are CSV files better than Excel?
When you open a CSV file in Microsoft Excel it looks the same👯♀️ as an Excel file with a .xls extension.
But both of them are different.
Excel files are good for storing complex🧠 data, but, whenever you want to import or export data from one program to another↔ CSV files are the best.
CSV files are extremely useful for website developers💻, eCommerce 💵businesses, and a lot more…
Ok, enough of the theory now let’s get our hands dirty by reading the CSV files.
Reading a CSV file in Python
You need to import the CSV module it’s built-in so no need to install it.
There are a lot of useful functions📚 and classes in this module.
Two are mainly significant ways to read a CSV file:
- reader() function
- DictReader() class
These are inside the CSV module.
You can imagine this as two 2⃣ checkpoints on your game’s roadmap. For reaching the first 🥇 checkpoint you’ll need to finish two ✌ levels. And from there to the second 🥈 checkpoint you’ll need to cross just 1 ☝ level.
Using the reader() Function: CheckPoint 1
LEVEL 1 – Reading using the reader() function
Let’s first download a sample CSV.
You can choose any here we’ll go with this one.
Source: https://people.sc.fsu.edu/~jburkardt/data/csv/csv.html
Now that you have the CSV file, open it using the with open block:
import csv
with open("addresses.csv") as csvObj:
pass
If you have no clue about how to open a file in python then read the previous post.
Next, inside the with block create a variable to store the content of this file. Then print it. This will make that variable a reader object.
import csv
with open("addresses.csv") as csvObj:
content = csv.reader(csvObj)#a reader object, It is an iterable
print(content)
Output:-
<_csv.reader object at 0x0000024FBF99DA80>
You’ll see that this function has returned an object.
This is an iterable object.
For now iterable means you can access its elements one after another using the next
method or loops. We will discuss it in detail in OOP.
To get the content inside of it iterate over it using a for loop or you can also use the next method:
import csv
with open("addresses.csv") as csvObj:
content = csv.reader(csvObj)
#To use next method
#print(next(content))
#next method will print 1 row at a time
for row in content:
print(row)
Output:-
['John', 'Doe', '120 jefferson st.', 'Riverside', ' NJ', ' 08075']
['Jack', 'McGinnis', '220 hobo Av.', 'Phila', ' PA', '09119']
['John "Da Man"', 'Repici', '120 Jefferson St.', 'Riverside', ' NJ', '08075']
['Stephen', 'Tyler', '7452 Terrace "At the Plaza" road', 'SomeTown', 'SD', ' 91234']
['', 'Blankman', '', 'SomeTown', ' SD', ' 00298']
['Joan "the bone", Anne', 'Jet', '9th, at Terrace plc', 'Desert City', 'CO', '00123']
Yeah, level 1 is done.🥳
LEVEL 2 – Reading with a different Delimiter
There’s a chance that the CSV file that you are working with has a delimiter(separator) other than a comma:
In that case, you’ll need to use the delimiter argument of the reader function:
import csv
with open("addresses_with_tab.csv") as csvObj:
content = csv.reader(csvObj, delimiter='|')
for row in content:
print(row)
Output:-
['John', 'Doe', '120 jefferson st.', 'Riverside', ' NJ', ' 08075']
['Jack', 'McGinnis', '220 hobo Av.', 'Phila', ' PA', '09119']
['John "Da Man"', 'Repici', '120 Jefferson St.', 'Riverside', ' NJ', '08075']
['Stephen', 'Tyler', '7452 Terrace "At the Plaza" road', 'SomeTown', 'SD', ' 91234']
['', 'Blankman', '', 'SomeTown', ' SD', ' 00298']
['Joan "the bone"| Anne', 'Jet', '9th| at Terrace plc', 'Desert City', 'CO', '00123']
Yeah, level 2 is also done.🥳
Checkpoint 1 has been reached.🎯
Let’s go ahead to checkpoint 2 now.🚶♂️
Using the DictReader() Class: CheckPoint 2
LEVEL 1
For this, we will use a different file.
You can use the same file but it doesn’t have headings for columns.
So the dictionary that you’ll get as output won’t make sense🥴. I’ll show you that as well.
Source: https://people.sc.fsu.edu/~jburkardt/data/csv/csv.html
To read it using the DictReader Class You need to just change reader to DictReader that’s it:
import csv
with open("nile.csv") as csvObj:
content = csv.DictReader(csvObj)
for row in content:
print(row)
#I am not showing the full output as it's too long.
Output:
{'Year': ' 1', ' "Flood"': ' 9.9974'}
{'Year': ' 2', ' "Flood"': ' 10.5556'}
{'Year': ' 3', ' "Flood"': ' 9.9014'}
.....
{'Year': ' 569', ' "Flood"': ' 10.4020'}
{'Year': ' 570', ' "Flood"': ' 10.3060'}
So the Dictreader class returns an iterable that has dictionaries as its elements unlike lists like in the reader function.
The file’s first row becomes the key for all the dictionaries and all the other data is filled according to the file.
Now if you would have done this with the addresses.csv file then the output would look like this:
{'John': 'Jack', 'Doe': 'McGinnis', '120 jefferson st.': '220 hobo Av.', 'Riverside': 'Phila', ' NJ': ' PA', ' 08075': '09119'}
{'John': 'John "Da Man"', 'Doe': 'Repici', '120 jefferson st.': '120 Jefferson St.', 'Riverside': 'Riverside', ' NJ': ' NJ', ' 08075': '08075'}
....
This output just doesn’t make sense that’s why I switched to a different file. I mean it says John is Jack, Doe is McGinnis, like seriously.😅
In the code, you just need to change nile to addresses if you wish to check the output.
And with that, you have cleared your second checkpoint too. Yeah.🎯
Now, let’s see how to write in a CSV file. New checkpoints on our road map.
Writing in a CSV file in python
Do you like traveling?🛫🛸
Do you also have plans to visit a lot of places or even for a world tour?🌏
Can be done for free? $
No, it’s not for any ads or product recommendations it’s just to say that we need some bits of financial 🗺💵 planning for this purpose.
And why not do that using our own favorite❣ python and CSV files? I know you can directly do that in a CSV but its time⌚ consuming and boring😪.
That’s why we’ll see how to write in a CSV file so you’re safe from hard work and boredom.
There are again 2 ✌ major ways(Two more checkpoints):
- writer() function
- DictWriter() class
To reach our third checkpoint 🎯 we’ll need to clear 3✌☝ levels. And to reach to the fourth one 🎯 we’ll need to clear 1 ☝ level.
You could have guessed and even tried what the code will look like by now.
Using the writer() Function: CheckPoint 3
LEVEL 1 – Writing using the writer() function
First, open a new file in w
mode. This can be an existing or a new file. If it’s an existing file then, it will be overwritten.
import csv
with open("places_to_travel.csv", "w") as csvObj:
Now create a writer object using the writer function.
import csv
with open("places_to_travel.csv", "w") as csvObj:
typer = csv.writer(csvObj)
Start adding as many rows as you want using the writerow
function on the writer object:
You would need to write a row in the form of a list.
import csv
with open("places_to_travel.csv", "w") as csvObj:
typer = csv.writer(csvObj)
#heading
typer.writerow(["City/Country", "Places To Travel"])
#rows
typer.writerow(["Paris", ["Eiffel Tower", "Louvre", "Montmartre"]])
typer.writerow(["Spain", ["Tenerife", "Alicante", "Barcelona"]])
typer.writerow(["France", ["Paris", "Marseille", "Lyon"]])
Now run the program and you’ll see a new file created or the existing file edited.
Output in the file:
City/Country,Places To Travel
Paris,"['Eiffel Tower', 'Louvre', 'Montmartre']"
Spain,"['Tenerife', 'Alicante', 'Barcelona']"
France,"['Paris', 'Marseille', 'Lyon']"
You’ll notice that this has left a line after every row. If you don’t want it then, just give an argument newline=''
in the open function.
with open("places_to_travel.csv", "w", newline = '') as csvObj:
With that, we cleared the first level🥳 to reach the third checkpoint.
LEVEL 2 – Writing multiple rows
Ok so now you might feel like we are calling the writerow
function a lot of times. You can avoid calling it again and again.
For that store all the lists of rows in another list. You know like a list of lists:
import csv
with open("places_to_travel.csv", "w") as csvObj:
row_list = [["City/Country", "Places To Travel"],
["Paris", ["Eiffel Tower", "Louvre", "Montmartre"]],
["Spain", ["Tenerife", "Alicante", "Barcelona"]],
["France", ["Paris", "Marseille", "Lyon"]]]
Now just create a writer object and write this using the writerows
function on it:
Remember its writerows
not writerow
!
import csv
with open("places_to_travel.csv", "w") as csvObj:
row_list = [["City/Country", "Places To Travel"],
["Paris", ["Eiffel Tower", "Louvre", "Montmartre"]],
["Spain", ["Tenerife", "Alicante", "Barcelona"]],
["France", ["Paris", "Marseille", "Lyon"]]]
typer = csv.writer(csvObj)
typer.writerows(row_list)
You’ll get the same output as in level 1 of this checkpoint.
🥳level 2 complete
Time for level 3.
LEVEL 3 – Writing with a different Delimiter
You might need or want to write a CSV file with a different delimiter(separator).
For that, you will need to give your desired delimiter inside the writer function:
import csv
with open("places_to_travel.csv", "w") as csvObj:
row_list = [["City/Country", "Places To Travel"],
["Paris", ["Eiffel Tower", "Louvre", "Montmartre"]],
["Spain", ["Tenerife", "Alicante", "Barcelona"]],
["France", ["Paris", "Marseille", "Lyon"]]]
typer = csv.writer(csvObj, delimiter="|")
typer.writerows(row_list)
Yeah,🥳 level 3 cleared.
And we have reached the third checkpoint🎯 as well.
And on the way to checkpoint 4.
Using the DictWriter() Class: CheckPoint 4
LEVEL 1
This class’s object is a writer that has the ability to map a dictionary to a row. I mean it will take your dictionary and convert it into a row of your CSV file.
For that you’ll need to first create a list of heads(field names) and then a list of dictionaries:
You can pass them separately to the writerow
function as well but you’ll need a list of heads.
import csv
with open("places_to_travel.csv", "w") as csvObj:
heads = ["City/Country", "Places To Travel"]
places_to_travel = [
{"City/Country":"Paris", "Places To Travel":["Eiffel Tower", "Louvre", "Montmartre"]},
{"City/Country":"Spain", "Places To Travel":["Tenerife", "Alicante", "Barcelona"]},
{"City/Country":"Greece", "Places To Travel":["The Acropolis", "Santorini", "Crete"]},
{"City/Country":"France", "Places To Travel":["Paris", "Marseille", "Lyon"]},
]
Now create a writer object using the DictWriter class and pass in file and heads(field names). Both are compulsory arguments.
And then write the header and rows:
import csv
with open("places_to_travel.csv", "w") as csvObj:
heads = ["City/Country", "Places To Travel"]
places_to_travel = [
{"City/Country":"Paris", "Places To Travel":["Eiffel Tower", "Louvre", "Montmartre"]},
{"City/Country":"Spain", "Places To Travel":["Tenerife", "Alicante", "Barcelona"]},
{"City/Country":"Greece", "Places To Travel":["The Acropolis", "Santorini", "Crete"]},
{"City/Country":"France", "Places To Travel":["Paris", "Marseille", "Lyon"]},
]
typer = csv.DictWriter(csvObj, heads)
typer.writeheader()
typer.writerows(places_to_travel)
Yeah, level 1 is complete.🥳
That’s it and you have arrived at checkpoint 4.🎯
Now just two more checkpoints.
Working with CSV files using the pandas library
There would be hardly any Pythonista who hasn’t at least heard the name of pandas library. It is super famous for data science, manipulation, and analysis.
I keep on telling how superb and amazing it is, and showcase all its uses but, for that, we will need a whole new category of posts.
We will discuss all of that and more but for now, know that whenever you are dealing with a CSV with a huge amount of data pandas is the best option.
But to use its functionality you can’t simply import it like the CSV library.
You need to install it first. You’ll need an internet connection for that.
So to install it open Windows PowerShell or cmd(command prompt)
In there just type:
pip install pandas
And wait. It takes time to install the library so please wait and don’t do anything while that time on your system.
And for the people who are following this on the android system just go to the pip tab in the app and then type in pandas and click install.
If you face any problems with this ask in the comment section below. You can also visit these official links of pandas and pip.
Now refresh your code editor(reopen if you can’t find a refresh option). And import pandas:
import pandas
In case you feel like its a long name to type every time you want to use its functionality, give it a pet name:
import pandas as pd
#you can write anything instead of pd
#you'll need to use that name throughout
#pd is used by most of the programmers
Now we can move on to checkpoint 5.
Reading CheckPoint 5
Reading a CSV file in python pandas is super easy. Just use the read_csv() function
import pandas as pd
travel = pd.read_csv("places_to_travel.csv")
print(travel)
Output:-
City/Country Places To Travel
0 Paris ['Eiffel Tower', 'Louvre', 'Montmartre']
1 Spain ['Tenerife', 'Alicante', 'Barcelona']
2 Greece ['The Acropolis'DataFrame, 'Santorini', 'Crete']
3 France ['Paris', 'Marseille', 'Lyon']
The read_csv
function returns a DataFrame datatype. It is a datatype just like an integer, string, list, dictionary, set, or tuple. We will discuss this in detail later.
The 0,1,2,3 are indexes of the DataFrame. You don’t need to get into detail right now.
We have reached our 5th checkpoint with this.
Let’s move on to the 6th and final checkpoint.
Writing CheckPoint 6
To write into a CSV file using the pandas we first need to convert the data into a DataFrame.
To convert the places_to_travel
list to a DataFrame:
import pandas as pd
travel_df = pd.DataFrame(places_to_travel)
Now we can write this DataFrame in the CSV file using the to_csv
function:
import pandas as pd
travel_df = pd.DataFrame(places_to_travel)
travel_df.to_csv("places_to_travel.csv")
Output in the CSV file:-
,City/Country,Places To Travel
0,Paris,"['Eiffel Tower', 'Louvre', 'Montmartre']"
1,Spain,"['Tenerife', 'Alicante', 'Barcelona']"
2,Greece,"['The Acropolis', 'Santorini', 'Crete']"
3,France,"['Paris', 'Marseille', 'Lyon']"
It has added 0,1,2,3 as we exported a DataFrame to the file.
You could have stored your data in a dictionary as well throughout:
places_to_travel2 = {"City/Country":["Paris", "Spain", "Greece", "France"],
"Places To Travel":[["Eiffel Tower", "Louvre", "Montmartre"],
["Tenerife", "Alicante", "Barcelona"],
["The Acropolis", "Santorini", "Crete"],
["Paris", "Marseille", "Lyon"]]}
This would have given the same results.
It has got n number of other functionalities. But it will all be covered when we will discuss the pandas library in detail.
Working with CSV on Android
Conclusion
So, in this post, we went through 6 checkpoints.
In this we understood, the working with CSV files in python with the help of 2 libraries namely:
- CSV, and
- Pandas
We covered reading data from a CSV file and writing data into a CSV file.
The official documentation of working with CSV files in python.
The official documentation of the pandas library.
Challenge🧗♀️
As a challenge, you need to store data of the two-player rock paper scissors game you made in the last post as a challenge in a CSV file.
It should look like this:-
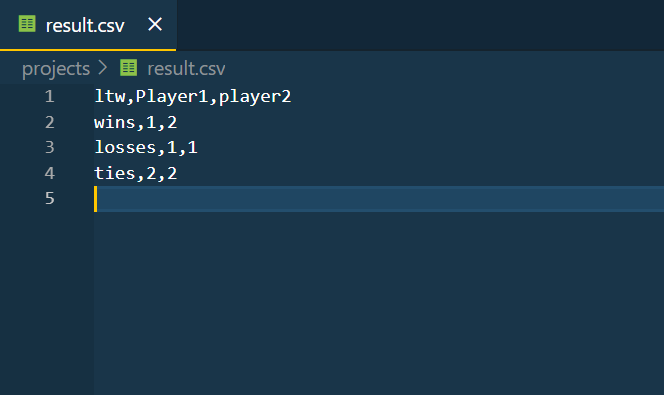
Type the fields(ltw, Player1, Player2) in the CSV. Just append the data. And yes, ltw = lost tie win.
This should be a pretty easy challenge for you. You would just need some extra variables and bits of modifications. That’s it.
Happy solving.
Go fast. I am waiting. Comment your answers below.
This was it for the post. Comment below suggestions if there and tell me whether you liked it or not. Do consider sharing this with your friends.
See you in the next post till then have a great time.😊
Admiring the hard work you put into your website and in depth information you present.
It’s awesome to come across a blog every once in a while
that isn’t the same old rehashed information. Excellent
read! I’ve saved your site and I’m adding your RSS feeds to my Google account.
Thanks a lot for your kind words…😊