Hello Pythonistas welcome back. Today we will continue our series CodeCraft: Building Skills One Project at a Time.
So let’s get started, the second project in this series is a Number Guessing Game.
Yes, we will together create a random number guessing game in Python.
Contents
Pre-requisites
You need to go through the previous project once. Just check out the source code here. If you understand this one you are good to go.
Why A Number Guessing Game?
Well, firstly cause it’s to create and play.
And, secondly, I provided a small list of challenges for you in the calculator, this one has error handling and running the project on loop features.
Thus, it is a good build-up for the previous project.
Approach To Create A Random Number Guessing Game In Python
Task: Build a game where the computer randomly selects a number, and the user has to guess it.
- Introduce the user to the rules.
- Generate a random number to be guessed.
- Initialize the attempt counter and a list to hold already guessed numbers.
- Let the user guess until the maximum guess limit is reached.
- If the user guesses the number correctly. Declare win.
- If the user has already guessed the number let him know.
- If the user has guessed a number less than the original one let him know and increase the attempt counter.
- If the user has guessed a number greater than the original one let him know and increase the attempt counter.
- Add the number to the guessed list.
- If the user runs out of guesses. Declare lost.
Step 1: Introduce the user to the rules.
# Step 1: Introduce the user to rules.
def introduce() -> None:
"""Introduces player to the rules of the game."""
print("You will get a total of 10 guesses.")
print("In 10 guesses you have to guess the correct no. between 1 to 50.")
print("READY???")
for count in range(1, 4):
print(count)
print("GO")
Here, I have simply created a function to introduce the player with the game.
Step 2: Generate a random number to be guessed.
import random
MIN_NUMBER = 1
MAX_NUMBER = 50
# Step 2: Generate a random number to be guessed.
def play_game():
num = random.randint(MIN_NUMBER, MAX_NUMBER)
Click For Explanation
First, we initialized the maximum and minimum values.
These values would define the range of numbers from which a random number could be generated.
Then, we have imported the random module of Python.
This module has a randint() method that returns a random number between the given range.
Step 3: Initialize the attempt counter and a list to hold already guessed numbers.
# Step 3: Initialize attempt counter and a list to hold already guessed numbers.
guess_list = []
attempt = 1
# Step 1 Extended to call the introduction.
introduce()
Step 4: Let the user guess until the maximum guess limit is reached.
# with the other macros.
MAX_ATTEMPTS = 10
# Step 4: Let the user guess until maximum guess limit is reached.
while attempt != (MAX_ATTEMPTS + 1):
# Handles the case where user entered a non-numeric value
try:
guess = int(input("Guess a no.:"))
except ValueError:
print("Enter a valid number!!")
continue
Click For Explanation
We have initialized a while loop that would run until the number of attempts crosses 11.
Then we used the try-catch mechanism, to ensure, that the user entered a valid number.
a. If the user guesses the number correctly. Declare win.
# Step 4: a. If the user guess the number correct. Declare win.
if guess == num:
print(f"\n\nYou got it right in {attempt} attempts.\nThe no. is {num}")
return
b. If the user has already guessed the number let him know.
# Step 4: b. If the user has already guessed the number let him know.
elif guess in guess_list:
print("You already guessed this no. before!")
c. If the user has guessed a number less than the original one let him know and increase the attempt counter.
# Step 4: c. If the user has guessed a number less than the original one let him know and increase the attempt counter.
elif guess > num:
print(f"The no. is less than {guess}")
attempt += 1
d. If the user has guessed a number greater than the original one let him know and increase the attempt counter.
# Step 4: d. If the user has guessed a number greater than the original one let him know and increase the attempt counter.
elif guess < num:
print(f"The no. is greater than {guess}")
attempt += 1
e. Add the number to the guessed list.
# Step 4: e. Add the number in the guessed list.
guess_list.append(guess)
Step 5: If the user runs out of guesses. Declare lost.
# Step 5: If the user runs out of guesses. Declare lost.
if attempt>=10:
print("GAME OVER")
print("The correct no. was",num)
if __name__ == "__main__":
play_game()
Click For Explanation
The if name == "main":
is to ensure that the code executes only when you run the current file.
Sample Output
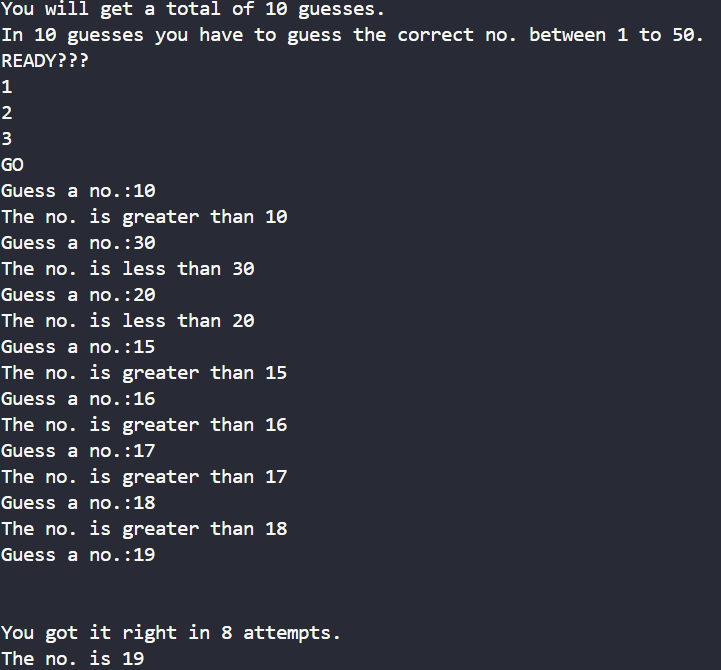
Full Source Code
Click For Code
import random
MIN_NUMBER = 1
MAX_NUMBER = 50
MAX_ATTEMPTS = 10
# Step 1: Introduce the user to rules.
def introduce() -> None:
"""Introduces player to the rules of the game."""
print("You will get a total of 10 guesses.")
print("In 10 guesses you have to guess the correct no. between 1 to 50.")
print("READY???")
for count in range(1, 4):
print(count)
print("GO")
# Step 2: Generate a random number to be guessed.
def play_game():
num = random.randint(MIN_NUMBER, MAX_NUMBER)
# Step 3: Initialize attempt counter and a list to hold already guessed numbers.
guess_list = []
attempt = 1
# Step 1 Extended
introduce()
# Step 4: Let the user guess until maximum guess limit is reached.
while attempt != (MAX_ATTEMPTS + 1):
# Handles the case where user entered a non-numeric value
try:
guess = int(input("Guess a no.:"))
except ValueError:
print("Enter a valid number!!")
continue
# Step 4: a. If the user guess the number correct. Declare win.
if guess == num:
print(f"\n\nYou got it right in {attempt} attempts.\nThe no. is {num}")
return
# Step 4: b. If the user has already guessed the number let him know.
elif guess in guess_list:
print("You already guessed this no. before!")
# Step 4: c. If the user has guessed a number less than the original one let him know and increase the attempt counter.
elif guess > num:
print(f"The no. is less than {guess}")
attempt += 1
# Step 4: d. If the user has guessed a number greater than the original one let him know and increase the attempt counter.
elif guess < num:
print(f"The no. is greater than {guess}")
attempt += 1
# Step 4: e. Add the number in the guessed list.
guess_list.append(guess)
# Step 5: If the user runs out of guesses. Declare lost.
if attempt>=10:
print("GAME OVER")
print("The correct no. was",num)
if __name__ == "__main__":
play_game()
Challenge For You!
Your challenge is to store the highest score that is when the player last guessed the number in the least number of attempts.
Video
Hope you had fun building this… See you in the next article till then, Happy Coding…
Create A Random Number Guessing Game In Python Create A Random Number Guessing Game In Python Create A Random Number Guessing Game In Python Create A Random Number Guessing Game In Python Create A Random Number Guessing Game In Python Create A Random Number Guessing Game In Python Create A Random Number Guessing Game In Python Create A Random Number Guessing Game In Python Create A Random Number Guessing Game In Python Create A Random Number Guessing Game In Python Create A Random Number Guessing Game In Python