Welcome to Day Twenty-One of my 21-day project series!
I have made a Real-Time Spelling Checker In Python.
This project is made so that we can get our spelling corrected when we are typing ridiculously fast.
Do read the experience…
Contents
Project Contents
This mini project is called A Real-Time Spelling Checker In Python
This is a very simple spelling checker that highlights the word written wrong. It is quite slow so might be frustrating at times.
It uses customtkinter, tkinter, re, and nltk library.
It is made completely using OOP concepts.
It looks like this:
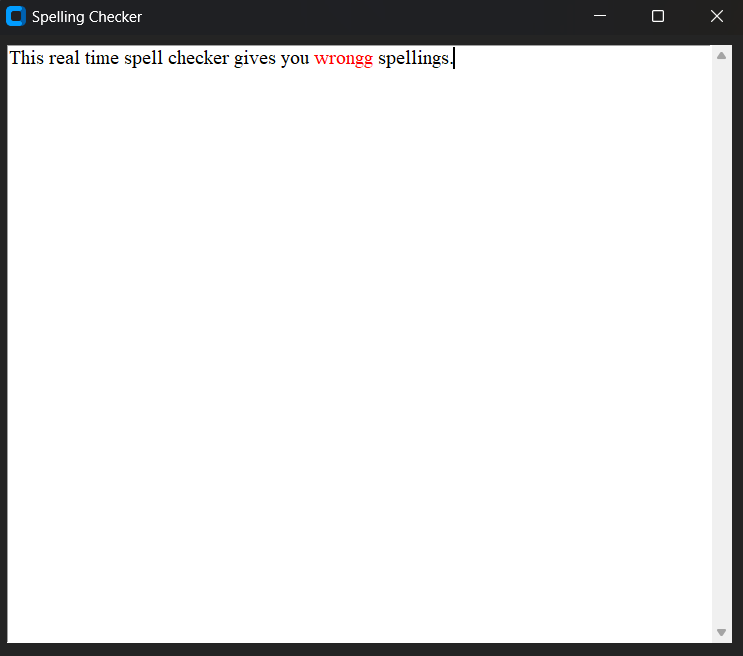
Tell me what should I improve in Real-Time Spelling Checker In Python.
I wouldn’t get into the step-by-step explanation of the code as the article becomes super long. (And I guess boring too.)
But I would post an explanatory article for it soon.
Full Source Code
Here’s the full source code of Real-Time Spelling Checker In Python:
# A GUI real time spell checker.
# for removing special characters
import re
# for GUI
from tkinter import scrolledtext
import tkinter as tk
from customtkinter import *
# for matching words
import nltk
from nltk.corpus import words
nltk.download("words")
class SpellingChecker(CTk):
def __init__(self):
super().__init__()
self.title("Spelling Checker")
self.geometry("600x500")
# Creating a widget to let user type
self.text_area = scrolledtext.ScrolledText(self,
wrap = tk.WORD,
width = 70,
height = 27,
font = ("Times New Roman",
15))
# To check the spellings whenever any key is released.
self.text_area.bind("<KeyRelease>", self.check)
self.text_area.grid(column = 0, pady = 10, padx = 10)
# keeping track of spaces.
self.old_spaces = 0
def check(self, event):
# getting whole content typed by user
content = self.text_area.get("1.0", tk.END)
# counting spaces
space_count = content.count(" ")
# checks spelling only if the space key was pressed.
if space_count != self.old_spaces: #checking if there are anymore spaces.
self.old_spaces = space_count #updating the new no. of spaces.
# removing any red highlights if there
for tag in self.text_area.tag_names():
self.text_area.tag_delete(tag)
# getting all the words
for word in content.split(" "):
# removinga any special characters if there
if re.sub(r"[^\w]", '', word.lower()) not in words.words():
# getting the starting position of incorrect word
position = content.find(word)
# marking wrong spelling red
self.text_area.tag_add(word, f"1.{position}", f"1.{position + len( word)}")
self.text_area.tag_config(word, foreground="red")
if __name__ == "__main__":
si = SpellingChecker()
si.mainloop()
Real-Time Spelling Checker In Python is well documented and well written it would be easy to understand. Here are the links to topics within the mini-project.
You can check them if you have any doubts or confusion:
Possible Improvements (That I’ll add after 21 days)
Here are some possible improvements in the Real-Time Spelling Checker In Python:
- Word Suggestions
- Custom Word Dictionary
- Performance Optimization
- Thesaurus Integration
- Dark Mode
- Auto-Correction
And a lot more…
Experience of the day
In case you don’t know this project is a part of the brand new series that I have started—#21days21projects in python. To read more about it you can check out this page.
And for those who already know here’s my experience of the day.
Finally, it’s day 21 and the end of the series.
Literally the first time in my life I have stuck to a challenge that is this long. I usually quit in 3-4 days. And max to max it lasts for 7 days.
But, this time it was different maybe cause I posted the challenge online.
I don’t know what made me get this far. But, it’s a satisfactory feeling for me.
Yeah, this is not the end, more like this is on the way…
Do let me know whether you liked this Real-Time Spelling Checker In Python. Also, let me know if there are any suggestions.
And most importantly how was your DAY 21?
Satisfactory?? Or Ambitious??
Well, I hope it was fun. And remember it’s perfectly awesome if you missed out. Continue to work from tomorrow.
And today just write one line of code as simple as making a variable or printing something.
Your suggestions are welcomed!
Happy coding…
Do let me know what you all will like to see in the future… Hope you got enriched in this challenge with me and explored a new side of yours…
Excellent post! Your insights on this topic are very valuable and have given me a new perspective. I appreciate the detailed information and thoughtful analysis you provided. Thank you for sharing your knowledge and expertise with us. Looking forward to more of your posts!
Thanks a lot!!