Hello Pythonistas welcome back. Today we are starting with a new series CodeCraft: Building Skills One Project at a Time.
https://www.calculator.net/create a simple calculator using Python dictionary.
Under this, we will be building projects in every article.
These projects would start from the very basics and will take your programming skills to the next level.
It is carefully crafted to make you a better programmer.
We will also discuss later how to find and participate in hackathons and win them in the future.
So let’s get started, the first project in this series is a simple calculator.
Yes, we will together create a simple calculator using Python Dictionary today.
Contents
Pre-requisites
- You need to have python installed in your system.
- You should know how to print Hello World in Python.
That’s it.
Why Calculator?
After you know how to print Hello World in any programming language the next obvious step is to learn about: inputs, variables, datatypes, and operators.
All of these could be easily understood using a calculator project.
Approach To Create A Simple Calculator Using Python Dictionary
Task: Create a calculator that performs basic arithmetic operations (addition, subtraction, multiplication, division).
- User Input: Prompt the user to enter two numbers and the operation they wish to perform.
- Perform Calculation: Based on the user’s input, perform the selected operation.
- Output the Result: Display the result of the operation to the user.
Flow To Create A Simple Calculator Using Python
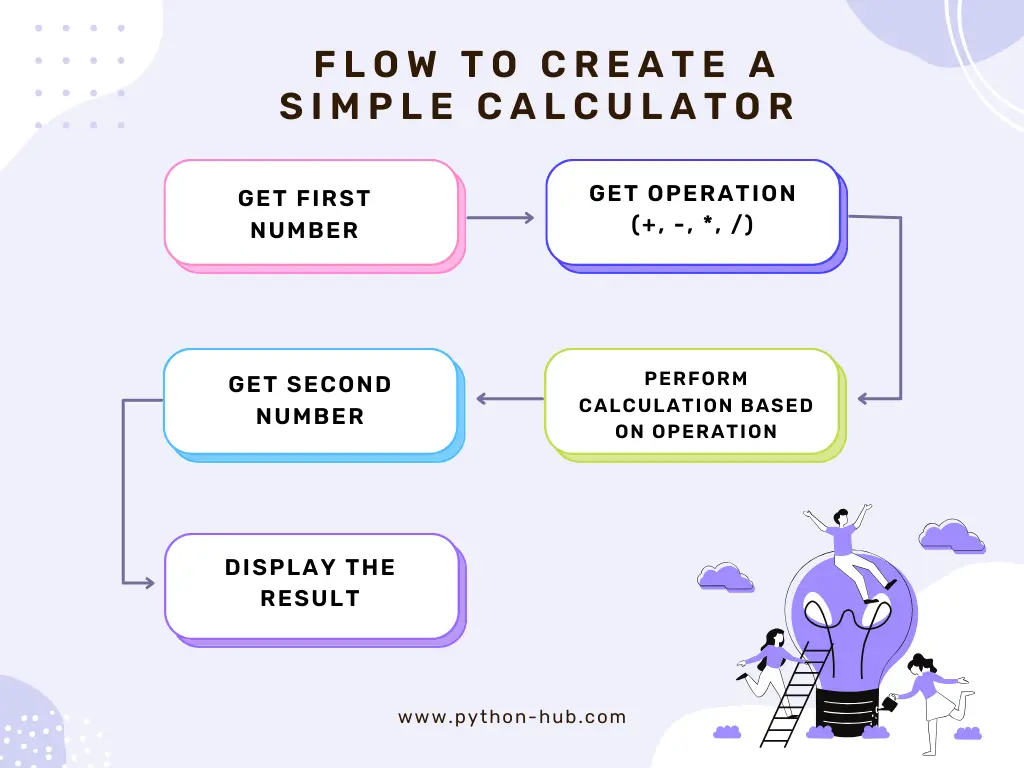
Step 1: Define A Function For Operations
A function is something that takes something as input, performs some operation, and provides some output.
In our case, we need to make a function that takes 2 values and an operator as input.
And provides the result of that arithmetic operation as output.
# Step 1: Define A Function For Operations
def calculate(n1:float, n2:float, operator:str) -> float:
operator_match = {
"+": n1+n2,
"-": n1-n2,
"x": n1*n2,
"/": n1/n2,
}
return operator_match[operator]
Click for Explanation
The function has taken 3 values as input.
Then, we have defined a dictionary to map the operators with specific operations.
A dictionary in python is a datatype that allows storing the data in a key-value format.
Using the key which in our case is the operator, we can access the value corresponding to it, which in this case, is the operation.
Now, we have simply returned: operator_match[operator]
.
For example: n1 = 10, n2 = 20, operator = “+”,
This return statement would go to the “+” operator in our operator_match dictionary and would return the addition of 10 and 20, that is 30.
Step 2: Get The Numbers And The Operator From The User
This function won’t work until we call it somewhere.
But to call this we function we need to provide it the values it takes.
We need to take these values as input from the user:
# Step 2: Get The Numbers And The Operator From The User
print("Select operation:")
print("1. Addition")
print("2. Subtraction")
print("3. Multiplication")
print("4. Division")
operator = input("Enter choice (+, -, x, /): ")
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
Click for Explanation
First, we have just displayed the operations that our project supports.
Then we take the operator as input.
Next, we take the two numbers as input.
The input function considers all the values as strings(a sequence of characters).
But we wanted the numbers to be decimal values.
That is why we converted the input numbers to float datatype.
Step 3: Perform The Calculation And Display The Result
Now, we just need to call the function and display the results.
# Step 3: Perform The Calculation And Display The Result
res = calculate(num1, num2, operator)
print(f"{num1} {operator} {num2} = {res}")
Click for Explanation
We have created a variable res to store the result provided by the calculate function.
Then we, are using an f-string to display the result of the operation in proper format.
Sample Output
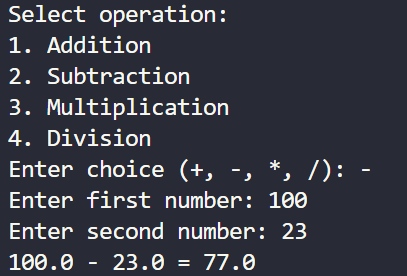
Full Source Code
Click Here
# Step 1: Define A Function For Operations
def calculate(n1:float, n2:float, operator:str) -> float:
operator_match = {
"+": n1+n2,
"-": n1-n2,
"x": n1*n2,
"/": n1/n2,
}
return operator_match[operator]
# Step 2: Get The Numbers And The Operator From The User
print("Select operation:")
print("1. Addition")
print("2. Subtraction")
print("3. Multiplication")
print("4. Division")
operator = input("Enter choice (+, -, *, /): ")
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
# Step 3: Perform The Calculation And Display The Result
res = calculate(num1, num2, operator)
print(f"{num1} {operator} {num2} = {res}")
Challenge For You!
Error Handling:
- Add error handling to manage invalid inputs (e.g., non-numeric inputs, invalid operation choices).
- Handle division by zero gracefully.
Extended Operations:
- Include additional operations like exponentiation, modulus, or square root.
Loop for Multiple Calculations:
- Allow the user to perform multiple calculations without restarting the program.